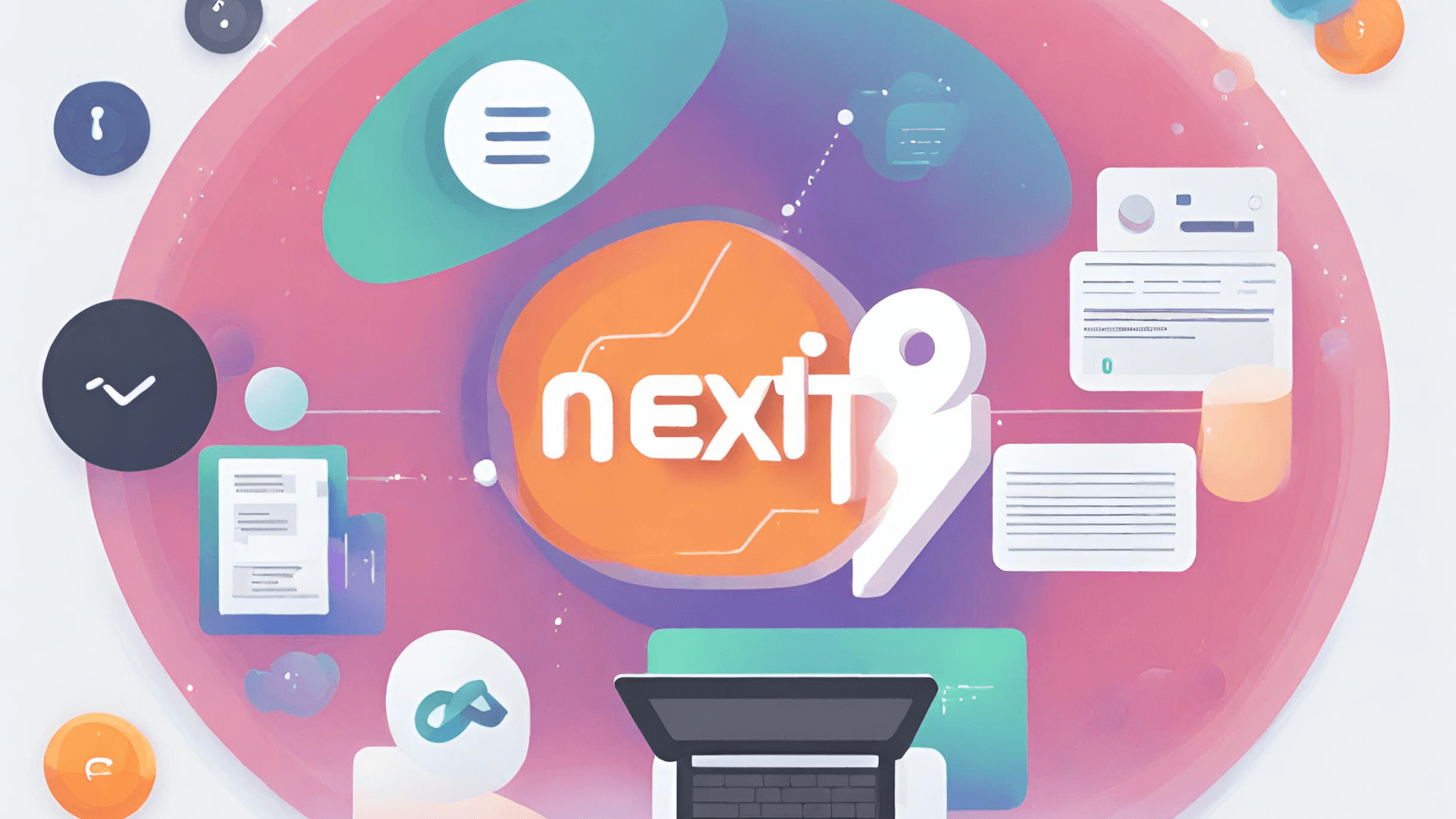
What is server-side rendering (SSR) in Next.js?
Server-Side Rendering (SSR) is a crucial aspect of modern web development, enhancing the performance and user experience of web applications. Next.js, a popular React framework, has gained widespread adoption due to its built-in support for SSR. In this article, we'll delve into the concept of Server-Side Rendering and explore how Next.js leverages it to create fast and dynamic web applications.
What is Server-Side Rendering (SSR)?
Server-Side Rendering refers to the technique of rendering web pages on the server rather than in the user's browser. Traditionally, web applications relied on Client-Side Rendering (CSR), where the browser was responsible for rendering the content. While CSR offers a smooth and dynamic user experience, it can result in slower initial page loads, especially on low-bandwidth or high-latency connections.
SSR addresses this issue by pre-rendering the content on the server and sending fully formed HTML pages to the client. This approach significantly improves the perceived performance and search engine optimization (SEO) of web applications.
The Role of Next.js in SSR
Next.js is a React framework that simplifies the process of implementing SSR in web applications. It seamlessly integrates server-side rendering, making it accessible to developers without requiring extensive configuration.
Key Features of Next.js SSR
Automatic Page Rendering:
Next.js automatically renders pages on the server, providing a faster initial page load. Developers can use the getServerSideProps
or getStaticProps
functions to fetch data and pre-render pages during the build process or at runtime.
// Example using getServerSideProps
export async function getServerSideProps(context) {
// Fetch data from an external API
const res = await fetch("https://api.example.com/data");
const data = await res.json();
// Pass data to the page component as props
return {
props: { data },
};
}
Dynamic Routing with SSR:
Next.js allows dynamic routing with SSR, enabling developers to create pages with dynamic parameters. This is achieved by creating files in the pages
directory with square brackets to denote dynamic segments.
// pages/[id].js
import { useRouter } from "next/router";
const DynamicPage = ({ data }) => {
const router = useRouter();
const { id } = router.query;
// Render page with dynamic data
return (
<div>
<h1>{`Data for ID: ${id}`}</h1>
{/* Render dynamic data here */}
</div>
);
};
export default DynamicPage;
Client-Side Navigation: While Next.js utilizes SSR for the initial page load, it seamlessly transitions to client-side navigation for subsequent page changes. This hybrid approach maintains the benefits of SSR while providing a smooth, interactive user experience.
import Link from "next/link";
const HomePage = () => {
return (
<div>
<h1>Home Page</h1>
<Link href="/about">
<a>About Page</a>
</Link>
</div>
);
};
export default HomePage;
Incremental Adoption: Developers can gradually adopt SSR in their applications with Next.js, allowing for incremental improvements without a complete rewrite. Existing React components can be integrated seamlessly into Next.js, and SSR can be selectively applied to specific pages.
Benefits of SSR in Next.js
-
Improved SEO: Search engines favor websites with pre-rendered content, as it allows them to crawl and index pages more effectively. Next.js SSR ensures that search engines receive fully formed HTML content, contributing to better SEO performance.
-
Enhanced Performance: By rendering pages on the server, Next.js reduces the burden on the client and provides faster initial page loads. This is especially beneficial for users on slower networks or less powerful devices.
-
Consistent User Experience: SSR in Next.js ensures a consistent user experience by delivering fully rendered pages to the client. Users see content immediately, reducing the time spent waiting for the page to load.
-
Social Media Sharing: When sharing links on social media platforms, the pre-rendered HTML content provided by SSR ensures that the shared content is accurately represented, including metadata and Open Graph tags.
Server-Side Rendering is a powerful technique for improving web application performance and user experience. Next.js, with its built-in support for SSR, simplifies the implementation process, making it accessible to a broader audience of developers. By leveraging SSR in Next.js, developers can create fast, SEO-friendly, and dynamically rendered web applications that meet the demands of modern web development. As the landscape of web technologies evolves, SSR continues to play a pivotal role in shaping the future of web applications.
Advanced SSR Techniques in Next.js
Data Fetching Strategies
Next.js provides different data fetching strategies for SSR, allowing developers to choose the most suitable approach based on their application's needs. The two primary strategies are getServerSideProps
and getStaticProps
. While getServerSideProps
fetches data on every request, getStaticProps
generates static pages at build time, offering a performance boost.
// Example using getStaticProps
export async function getStaticProps() {
// Fetch data from an external API
const res = await fetch("https://api.example.com/data");
const data = await res.json();
// Pass data to the page component as props
return {
props: { data },
};
}
API Routes
Next.js allows the creation of API routes, which can be used to handle server-side logic and fetch data. This approach separates the concerns of rendering pages and handling data, providing a clean and modular architecture.
// pages/api/data.js
export default function handler(req, res) {
// Fetch data from an external API
const data = // ...
// Send data as JSON response
res.status(200).json({ data });
}
Server Middleware
Next.js supports the use of server middleware, allowing developers to customize the server behavior. This is particularly useful for implementing authentication, logging, or other server-side logic.
// Example of server middleware
const middleware = (req, res, next) => {
// Custom logic here
next();
};
module.exports = middleware;
Challenges and Considerations
While SSR in Next.js offers numerous advantages, it's essential to be aware of potential challenges:
-
Server Load: Rendering pages on the server can increase the server load, especially in applications with a high volume of concurrent users. Proper server scaling and optimization are crucial for maintaining performance.
-
Complexity in Development: Implementing SSR introduces additional complexity, particularly when dealing with asynchronous data fetching and server-side logic. Careful planning and adherence to best practices are necessary to mitigate potential issues.
Future Trends
As the web development landscape evolves, SSR remains a critical aspect of building performant and scalable applications. The rise of Jamstack architecture and the increased emphasis on user experience and SEO make SSR even more relevant. Next.js is likely to continue evolving, incorporating new features and optimizations to further streamline the SSR process.
Server-Side Rendering in Next.js is a powerful tool for web developers seeking to create high-performance, SEO-friendly applications. Its seamless integration with React and its flexible data fetching strategies make it accessible to developers of varying skill levels. By understanding the core concepts and advanced techniques of SSR in Next.js, developers can unlock the full potential of this framework and deliver exceptional user experiences on the web. As the technology landscape advances, SSR in Next.js remains at the forefront of web development practices, shaping the way we build and deploy modern web applications.