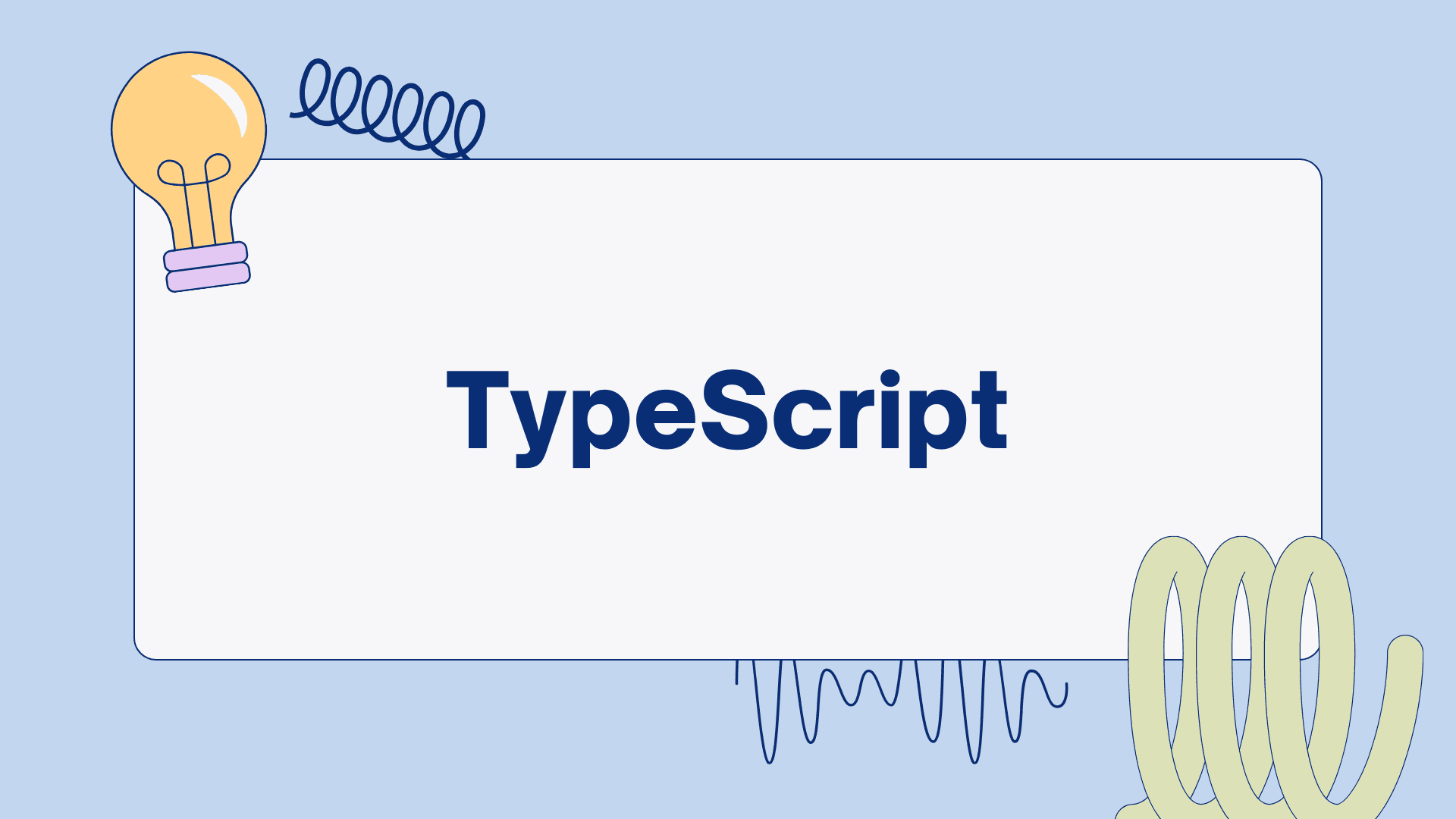
Can use TypeScript with Next.js?
Next.js has emerged as a popular React framework for building robust web applications, offering developers a powerful set of features to enhance their development experience. One of the key strengths of Next.js is its flexibility, which allows developers to seamlessly integrate TypeScript into their projects. In this article, we'll explore the benefits of using TypeScript with Next.js and guide you through the process of setting up a Next.js project with TypeScript.
Understanding TypeScript and Next.js
TypeScript Overview
TypeScript is a statically typed superset of JavaScript that adds optional static typing to the language. It allows developers to catch type-related errors early in the development process, offering improved code quality and maintainability. By introducing a type system, TypeScript enhances code readability, facilitates collaboration, and provides better tooling support.
Next.js Overview
Next.js is a React framework that simplifies the process of building server-rendered React applications. It offers features such as automatic code splitting, server-side rendering, and simplified routing, making it an attractive choice for developers seeking efficient and scalable web development solutions.
Setting Up a Next.js Project with TypeScript
Creating a New Next.js Project
To start using TypeScript with Next.js, you can create a new Next.js project using the following command:
npx create-next-app my-next-app
Replace "my-next-app" with your desired project name. This command sets up a basic Next.js project with default configurations.
Adding TypeScript
Next, navigate to the project directory and install the required TypeScript dependencies:
cd my-next-app
npm install --save-dev typescript @types/react @types/node
This installs TypeScript and the necessary type definitions for React and Node.js.
Configuring TypeScript
Create a tsconfig.json
file in the project root to configure TypeScript. Here's a basic example:
{
"compilerOptions": {
"target": "esnext",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"moduleResolution": "node",
"jsx": "preserve",
"lib": ["dom", "es2018"]
}
}
This configuration file specifies the TypeScript compiler options for your project.
Updating File Extensions
Change the file extensions of your React components to .tsx
instead of .js
or .jsx
. TypeScript recognizes these files as containing both JavaScript and JSX.
Benefits of Using TypeScript with Next.js
Improved Code Quality
TypeScript's static typing helps catch potential bugs during development, leading to more robust and reliable code.
Enhanced Developer Experience
TypeScript provides better tooling support in modern code editors, offering features like auto-completion, type inference, and inline documentation.
Code Readability and Maintainability
TypeScript's type annotations improve code readability and make it easier for developers to understand the structure of the codebase.
Seamless Integration
Next.js has excellent support for TypeScript, allowing developers to seamlessly integrate it into their projects without significant configuration hassles.
Advanced TypeScript Features in Next.js
Type Safety in API Routes
Next.js supports API routes, which enable you to build serverless functions easily. TypeScript adds an extra layer of safety by allowing you to define types for your API route parameters and responses. This ensures that your API contracts are clear and helps catch errors early in the development process.
// pages/api/users/[id].ts
import { NextApiRequest, NextApiResponse } from "next";
type Data = {
id: string;
name: string;
};
export default (req: NextApiRequest, res: NextApiResponse<Data>) => {
const {
query: { id },
} = req;
const user = {
id,
name: "John Doe",
};
res.status(200).json(user);
};
Generics for Reusable Components
TypeScript generics can be used to create reusable components with flexible types. This is particularly beneficial when developing shared components that can adapt to different data structures.
// components/GenericList.tsx
interface GenericListProps<T> {
items: T[];
renderItem: (item: T) => React.ReactNode;
}
function GenericList<T>({ items, renderItem }: GenericListProps<T>) {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{renderItem(item)}</li>
))}
</ul>
);
}
Strict Mode
Enabling TypeScript's strict mode ("strict": true
in tsconfig.json
) ensures a higher level of type safety by enabling strict null checks, strict function types, and other strict checks. This can help catch potential issues before they become runtime errors.
// tsconfig.json
{
"compilerOptions": {
"strict": true
}
}
Common Challenges and Solutions
Third-Party Libraries
While many popular libraries have TypeScript support, some may not provide type declarations out of the box. In such cases, you can install community-contributed type definitions using the @types
scope, or create custom type declarations for the library.
npm install --save-dev @types/library-name
Configuration Complexity
As your project grows, managing a complex tsconfig.json
file may become challenging. Tools like tsc --init
and tsconfig.json
generators can assist in creating and maintaining a well-organized TypeScript configuration.
Integrating TypeScript with Next.js goes beyond basic type checking, providing a foundation for a more robust and maintainable codebase. The advanced features and strong typing capabilities empower developers to build scalable applications with confidence. While there may be a learning curve, the benefits of improved code quality, developer experience, and maintainability make TypeScript a valuable addition to your Next.js projects. As the TypeScript and Next.js ecosystems continue to evolve, developers can expect even more powerful features and seamless integration in the future.
By combining the power of TypeScript and Next.js, developers can enjoy the benefits of static typing while building scalable and efficient React applications. The integration process is straightforward, and the resulting codebase is more resilient and maintainable. Whether you are starting a new project or considering migrating an existing one, leveraging TypeScript with Next.js is a wise choice that can significantly enhance your development workflow.