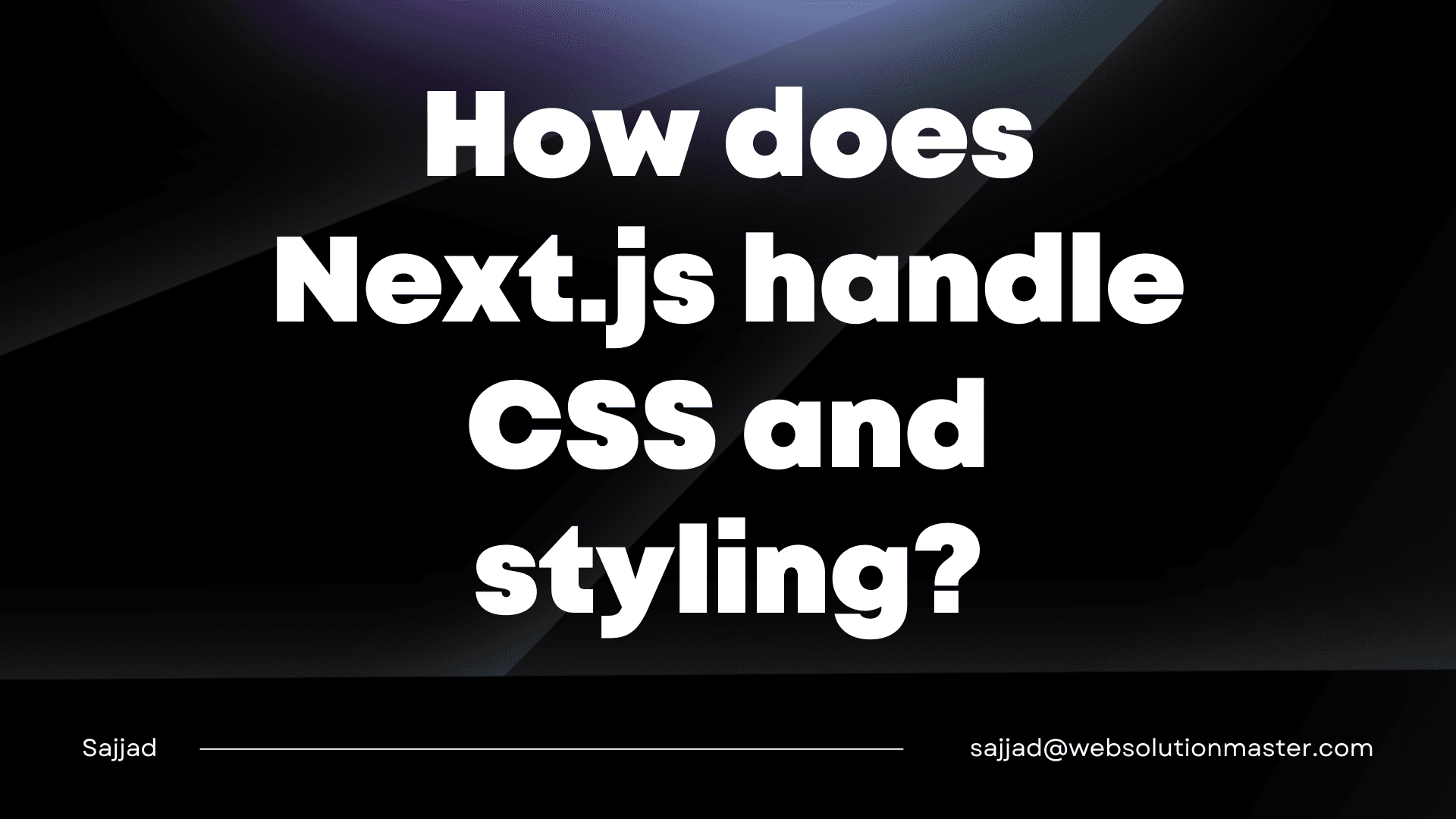
How does Next.js handle CSS and styling?
Next.js is a powerful and popular React framework that simplifies the process of building modern web applications. One of the key aspects of creating a visually appealing and responsive web application is styling. In the case of Next.js, the framework provides several approaches to handle CSS and styling, catering to various preferences and requirements of developers.
CSS Modules in Next.js
CSS Modules offer a way to scope styles to a particular component or module, preventing global styles from affecting other parts of the application. Next.js supports CSS Modules out of the box, making it a straightforward and efficient way to handle styling.
How to use CSS Modules in Next.js
-
File Naming Convention:
- Stylesheets using CSS Modules should follow a specific naming convention, such as
style.module.css
. The.module.css
extension indicates that it is a CSS Module.
- Stylesheets using CSS Modules should follow a specific naming convention, such as
-
Importing Styles:
-
When importing styles into a React component, the import statement should include the styles as an object. For example:
import styles from "./style.module.css"; function MyComponent() { return <div className={styles.myClass}>Hello, Next.js!</div>; }
-
By using CSS Modules, you ensure that styles are scoped to the respective components, avoiding unintended side effects due to global styles.
Styled JSX
Styled JSX is a unique styling approach introduced by Next.js. It allows developers to write component-specific styles directly within the component file, offering a seamless integration of CSS with React components.
Key features of Styled JSX
-
Scoped Styles:
- Styles defined using Styled JSX are scoped to the component, preventing conflicts with styles in other components.
-
Dynamic Styles:
- Styled JSX supports dynamic styles by allowing the use of JavaScript expressions within the styles. This is particularly useful for responsive designs or conditionally applying styles.
Example of Styled JSX
function MyComponent() {
const textColor = "blue";
return (
<div>
<p className="my-text">Styled JSX Example</p>
{/* Dynamic styles */}
<style jsx>{`
.my-text {
color: ${textColor};
}
`}</style>
</div>
);
}
Global Styles
In addition to component-specific styling, Next.js supports the use of global styles for applying consistent styles across the entire application.
How to include global styles in Next.js
-
Create a Global Stylesheet:
- Create a global stylesheet, for example,
global.css
in thestyles
directory.
- Create a global stylesheet, for example,
-
Import Global Styles:
- Import the global stylesheet in the
pages/_app.js
file. This file is a special file in Next.js that runs on every page.
// pages/_app.js import "../styles/global.css"; function MyApp({ Component, pageProps }) { return <Component {...pageProps} />; } export default MyApp;
- Import the global stylesheet in the
With this setup, the global styles will be applied consistently across all pages in the Next.js application.
CSS-in-JS Libraries Integration
For developers who prefer using CSS-in-JS libraries like Styled Components or Emotion, Next.js offers seamless integration. These libraries allow developers to write styles directly in their JavaScript code, making it easier to manage component styles and logic in a single file.
Integrating Styled Components
-
Install the library:
-
Use npm or yarn to install Styled Components.
npm install styled-components
-
-
Usage in Next.js:
-
Import and use Styled Components in your components.
// Example using Styled Components import styled from "styled-components"; const StyledDiv = styled.div` color: red; `; function MyComponent() { return <StyledDiv>Hello, Styled Components!</StyledDiv>; }
-
This integration provides a powerful solution for managing styles and components in a more cohesive manner.
Enhancing Styling in Next.js Beyond the Basics
As we delve deeper into the world of styling in Next.js, it's essential to explore advanced techniques and tools that can enhance the development experience and provide more control over the application's aesthetics. In this continuation, we will discuss additional features and strategies for handling CSS and styling in Next.js.
CSS Preprocessors
Next.js supports popular CSS preprocessors like Sass and Less, allowing developers to leverage advanced features such as variables, mixins, and nested styles. Using a preprocessor can enhance code organization and maintainability.
Integrating Sass
-
Install the Sass package:
-
Use npm or yarn to install the
sass
package.npm install sass
-
-
Create a Sass file:
- Create a Sass file, for example,
styles/mystyles.scss
.
- Create a Sass file, for example,
-
Import Sass in your components:
-
Import the Sass file directly in your components.
// Example using Sass import "../styles/mystyles.scss"; function MyComponent() { return <div className="my-sass-class">Hello, Sass!</div>; }
-
By incorporating preprocessors like Sass, developers can write more modular and maintainable stylesheets, improving the overall structure of the codebase.
CSS-in-JS Libraries: Tailwind CSS
Tailwind CSS has gained popularity for its utility-first approach, offering a vast set of pre-defined classes that can be applied directly in the markup. Next.js seamlessly integrates with Tailwind CSS, providing a quick and efficient way to build responsive and visually appealing user interfaces.
Integrating Tailwind CSS
-
Install Tailwind CSS and its dependencies:
-
Install
tailwindcss
,postcss
, andautoprefixer
using npm or yarn.npm install tailwindcss postcss autoprefixer
-
-
Initialize Tailwind CSS:
-
Create a configuration file and initialize Tailwind CSS.
npx tailwindcss init -p
-
-
Configure styles in your components:
-
Use Tailwind CSS classes directly in your components.
// Example using Tailwind CSS function MyComponent() { return ( <div className="bg-blue-500 text-white p-4">Hello, Tailwind CSS!</div> ); }
-
Tailwind CSS can significantly speed up the styling process, especially for developers who prefer a utility-first approach to building user interfaces.
Optimizing for Production
Efficient styling is crucial for optimal performance in production environments. Next.js provides features to optimize CSS and minimize its impact on the application's loading time.
CSS Minification
-
Enable CSS Minification:
- By default, Next.js minifies CSS files in production, reducing their size for faster loading times.
-
Extracting Critical CSS:
- Next.js allows the extraction of critical CSS to improve initial page load performance. This ensures that only essential styles are loaded initially, and the remaining styles are loaded asynchronously.
// pages/_app.js
import "../styles/globals.css";
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />;
}
export default MyApp;
Optimizing CSS for production, developers can ensure that their Next.js applications deliver a smooth and responsive user experience.
Handling CSS and styling in Next.js goes beyond the basics, offering a rich set of features and integrations that cater to diverse developer preferences and project requirements. Whether you choose CSS Modules, Styled JSX, global styles, preprocessors, or utility-first approaches like Tailwind CSS, Next.js empowers developers with the flexibility and tools needed to create visually stunning and performant web applications. As you explore the various styling options, consider the specific needs of your project and team to make informed decisions that lead to a more efficient and enjoyable development experience.
Next.js offers a flexible and comprehensive approach to handling CSS and styling in web applications. Whether you prefer CSS Modules, Styled JSX, global styles, or CSS-in-JS libraries, Next.js accommodates various preferences, enabling developers to choose the styling approach that best fits their project requirements. By providing these options, Next.js empowers developers to create visually appealing and maintainable web applications with ease.