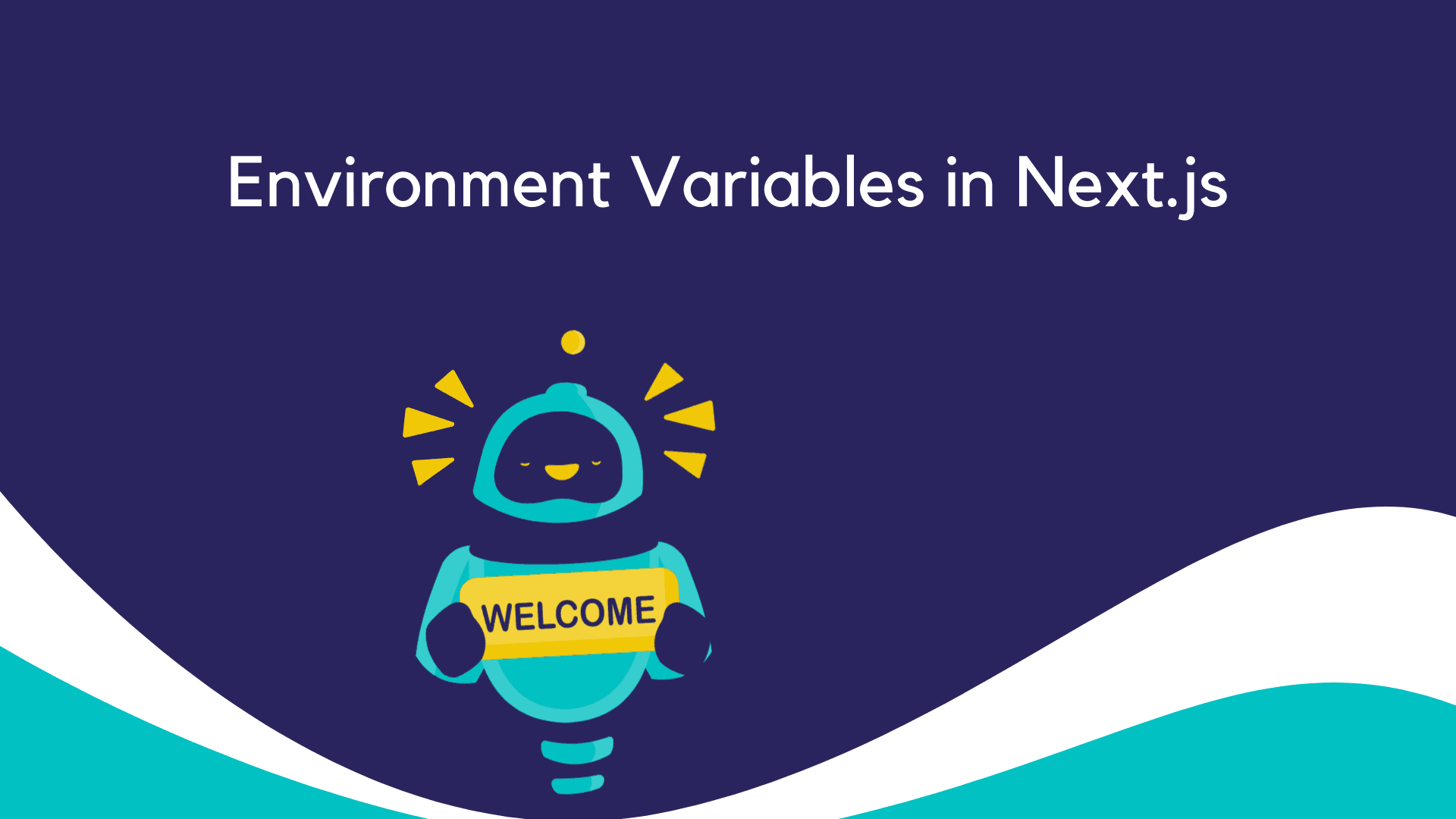
How to Use Environment Variables in Next.js
In modern web development, managing environment variables is crucial for keeping your application's sensitive data secure and your configurations flexible. For developers using Next.js, a popular React framework, understanding how to effectively use environment variables is essential. This article delves into the methods and best practices for handling environment variables in Next.js applications.
Understanding Environment Variables
Environment variables are key-value pairs used to store configuration settings and sensitive information, such as API keys, database passwords, and URLs, outside of your application code. They enable you to change the behavior of your application without altering the codebase, making your application more secure and adaptable to different environments (development, testing, production, etc.).
Setting Up Environment Variables in Next.js
Next.js supports environment variables natively, making it straightforward to implement them in your project. Here's how you can set up and use environment variables in a Next.js application.
Creating Environment Files
Start by creating environment files in your project's root directory. Next.js automatically supports the .env.local
, .env.development
, .env.test
, and .env.production
files. For example:
.env.local
: Variables for your local development environment..env.development
: Variables specifically for the development stage..env.production
: Variables for the production environment.
Adding Environment Variables
Inside your environment files, add your variables in the format KEY=VALUE
. For example:
API_KEY=your_api_key_here
DATABASE_URL=your_database_url_here
Prefixing Variables for the Browser
If you want to expose a variable to the browser, you must prefix it with NEXT_PUBLIC_
. This prefix tells Next.js to bundle this variable and make it available in the browser. For example:
NEXT_PUBLIC_API_URL=https://api.example.com
Accessing Environment Variables in Your Code
You can access environment variables in your Next.js application using process.env
. For example:
const apiKey = process.env.API_KEY;
const publicApiUrl = process.env.NEXT_PUBLIC_API_URL;
Remember that only variables prefixed with NEXT_PUBLIC_
are accessible from the client-side code.
Best Practices and Considerations
-
Security: Never expose sensitive data like database passwords or secret keys on the client side. Only use the
NEXT_PUBLIC_
prefix for variables that are safe to be exposed. -
Version Control: Do not commit your
.env
files to version control, especially if they contain sensitive information. Instead, add them to your.gitignore
file. You can create a.env.example
file with dummy values as a reference for other developers. -
Default Values: You can set default values for your environment variables in your code in case they are not set in the environment files.
-
Server-Side Variables: Remember that variables without the
NEXT_PUBLIC_
prefix are only available in the Node.js environment. This means they are only accessible during server-side rendering and in API routes. -
Runtime Configuration: For variables that need to change at runtime (after the build phase), consider using a different approach, as Next.js environment variables are embedded into the build and cannot be changed at runtime.
-
Loading Environment Variables: Next.js loads environment variables automatically at build time. However, if you add new variables after the build, you'll need to rebuild your application for the changes to take effect.
-
Avoiding Hardcoded Values: Ensure that you don't have hardcoded values in your application that can be better managed through environment variables. This makes your application more flexible and easier to configure across different environments.
Advanced Usage and Troubleshooting
Dynamic Environment Variables
In some scenarios, you might need to adjust environment variables dynamically based on certain conditions. This can be done by modifying the environment files before running the Next.js application or by using a custom server configuration.
-
Modifying Environment Files: You can write scripts that alter your
.env
files before starting your application. This can be useful in containerized environments like Docker. -
Custom Server Configuration: If you are using a custom server with Next.js, you can dynamically set environment variables in your server script. However, remember that this approach may affect the universal (isomorphic) nature of your Next.js application.
Local Development and Testing
When working in a team, ensure that each team member knows the required environment variables. A common practice is to maintain a .env.example
file with all the necessary environment variables listed (without their actual values). Team members can copy this file into their own .env.local
file and populate it with their values.
For automated tests, you can set environment variables in your testing setup. Frameworks like Jest allow you to set environment variables in the configuration file or before running tests.
Troubleshooting Common Issues
-
Variables Not Recognized: If your environment variables are not being recognized, ensure that they are defined in the correct
.env
file and that you've restarted your development server after adding them. -
Sensitive Data Exposure: Accidentally exposing sensitive data is a common pitfall. Double-check that any sensitive keys or secrets are not prefixed with
NEXT_PUBLIC_
. -
Build Time vs. Runtime: Understanding the difference between build-time and runtime is crucial in Next.js. If you set an environment variable after the application is built, it won't be included in the build. For variables that need to change after the build, consider other strategies like fetching configuration from an API.
Using Environment Variables with Third-Party Services
If your Next.js application interacts with third-party services, you'll likely need to use environment variables to store API keys, service URLs, and other configuration details. Ensure these variables are properly secured and only included in server-side or secure client-side code as necessary.
Environment Variables in Static Exports
If you’re using next export
to generate a static website, be aware that environment variables are set at build time. Since there's no server rendering, all variables need to be embedded at build time or managed through client-side JavaScript.
Debugging
For debugging purposes, you can log environment variables in your Next.js application. However, be cautious not to log any sensitive information, especially in production environments.
Incorporating environment variables into your Next.js application is a key part of creating secure, flexible, and maintainable web applications. By understanding how to set, access, and manage these variables, and by being aware of common pitfalls and best practices, you can effectively manage application configurations across different environments. As you continue to develop with Next.js, keep exploring and refining your approach to environment variable management to ensure the security and efficiency of your applications.
Conclusion
Using environment variables in Next.js is a straightforward but powerful way to manage your application's configuration and sensitive data. By following these guidelines and best practices, you can ensure that your Next.js application is secure, flexible, and easy to maintain across different environments. Remember to keep sensitive information out of the client-side code and to use the NEXT_PUBLIC_
prefix responsibly. With these techniques, you'll be able to harness the full potential of environment variables in your Next.js projects.