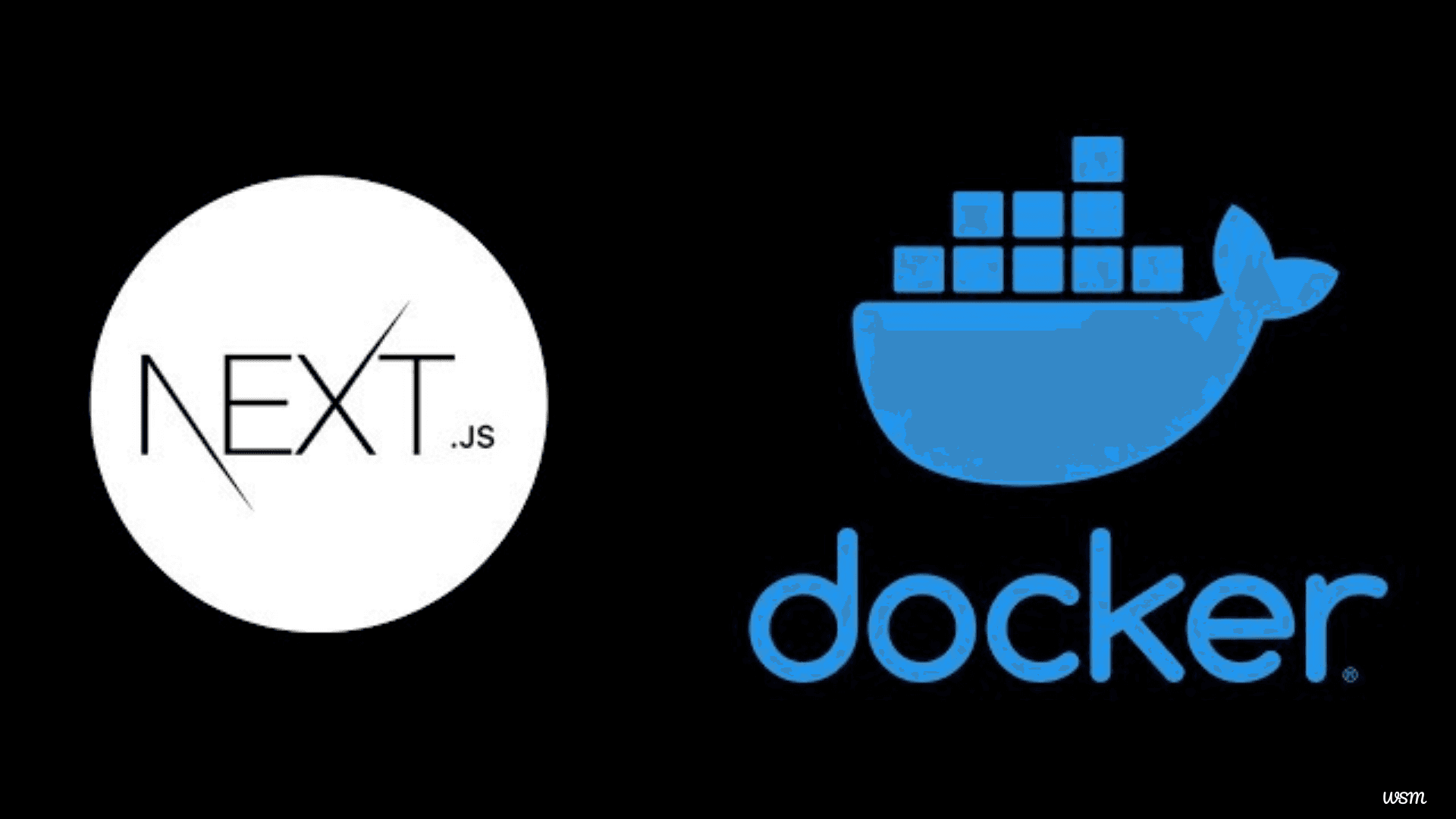
How to use Docker to containerize a Next.js app?
Containerization has become a fundamental practice in modern software development. By containerizing your Next.js application with Docker, you can ensure consistency across development, staging, and production environments. This approach allows developers to eliminate the infamous "it works on my machine" problem by creating isolated environments for applications. In this guide, I’ll walk you through the entire process of containerizing a Next.js application, from creating a Dockerfile to running your app in a container, ensuring your application is portable and deployable across any infrastructure.
In the ever-evolving landscape of web development, two technologies have gained significant traction: Docker and Next.js. Docker, a platform for developing, shipping, and running applications in containers, has revolutionized the way developers package and deploy software. Next.js, on the other hand, is a powerful React framework that enables developers to build server-side rendered and statically generated web applications with ease.
When combined, these technologies offer a robust solution for creating scalable, efficient, and easily deployable web applications. Docker's containerization approach ensures consistency across different environments, while Next.js provides a seamless development experience with its built-in features like automatic code splitting and optimized performance.
In this comprehensive guide, we'll explore how to harness the power of Docker to containerize a Next.js application. We'll walk you through the entire process, from setting up Docker on your system to deploying your containerized Next.js app, and share best practices along the way.
Why Use Docker for Your Next.js App?
Docker allows you to package your application and its dependencies into a container. This ensures that your app behaves the same, no matter where it runs. For Next.js, which is a React-based framework for server-rendered and statically exported apps, Docker provides benefits such as:
- 🛠️ Portability: Run your app on any system with Docker installed, whether it’s a developer's machine, a CI/CD pipeline, or a production server.
- 🔒 Isolation: Keep dependencies and configurations separate from the host system, reducing conflicts and potential security vulnerabilities.
- 📈 Scalability: Simplify the process of deploying your app to multiple servers or scaling it horizontally in response to increased user demand.
- 🚀 Streamlined Deployment: Docker containers are lightweight and can be easily orchestrated with tools like Kubernetes, making them ideal for cloud-native deployments.
Leveraging Docker, you create a standardized workflow that reduces bugs and deployment issues, saving time and resources.
Why containerize a Next.js app?
Containerizing your Next.js application offers numerous benefits that can significantly enhance your development workflow and deployment process. Here are some compelling reasons to consider containerization:
Consistency across environments: Docker containers encapsulate your application and its dependencies, ensuring that it runs consistently across different environments, from development to production. This eliminates the infamous "it works on my machine" problem and reduces the likelihood of environment-related issues.
Improved scalability: Containers are lightweight and can be quickly spun up or down, making it easier to scale your application horizontally. This is particularly beneficial for Next.js apps that may need to handle varying levels of traffic.
Simplified deployment: With Docker, you can package your Next.js app and all its dependencies into a single container image. This makes deployment a breeze, as you can easily ship and run the same container across different hosting platforms or cloud providers.
Isolation and security: Containers provide a level of isolation between your application and the host system, enhancing security. This isolation also allows you to run multiple applications on the same host without conflicts between dependencies or configurations.
Version control for infrastructure: Dockerfiles and Docker Compose files can be versioned alongside your application code, giving you better control over your infrastructure and making it easier to roll back to previous versions if needed.
Containerizing your Next.js app, you're not only streamlining your development process but also setting yourself up for a more efficient and manageable production environment.
Prerequisites
Before diving into the tutorial, ensure you have the following:
- 🐋 Docker installed on your machine. If not, follow the official installation guide.
- 🟢 Node.js and npm (or Yarn) installed, as you’ll need them to run and build the Next.js app locally.
- 📚 Basic knowledge of Docker commands, Dockerfile syntax, and the fundamentals of Next.js development.
Additionally, ensure you have a text editor or IDE such as VS Code installed to write the necessary configuration files and scripts.
Setting Up a Next.js Project
If you already have a Next.js project, you can skip this step. Otherwise, let’s create a simple Next.js app to work with:
npx create-next-app@latest my-nextjs-app
cd my-nextjs-app
Start the development server to verify the app works:
npm run dev
Visit http://localhost:3000
in your browser to see the default Next.js welcome page. This step ensures that your development environment is properly configured before proceeding with Docker.
Writing the Dockerfile
A Dockerfile is a script that defines how the Docker image for your app will be built. It’s the blueprint for creating an efficient and functional container. Let’s create a Dockerfile
in the root of your project.
Example Dockerfile
# Stage 1: Build the application
FROM node:18 AS builder
# Set working directory
WORKDIR /app
# Copy package.json and package-lock.json
COPY package*.json ./
# Install dependencies
RUN npm install
# Copy the rest of the application code
COPY . .
# Build the Next.js app
RUN npm run build
# Stage 2: Serve the application
FROM node:18
# Set working directory
WORKDIR /app
# Copy built files from the builder stage
COPY --from=builder /app .
# Expose the Next.js default port
EXPOSE 3000
# Start the application
CMD ["npm", "start"]
Explanation of Each Stage
- 🛠️ Stage 1 (Builder): This stage uses the official Node.js image to install dependencies and build the Next.js app. By using a multi-stage build, we optimize the final image size by excluding development dependencies and intermediate files from the runtime environment.
- 🖥️ Stage 2 (Runtime): The final image contains only the files necessary to run the built app, resulting in a smaller and more efficient container.
By structuring the Dockerfile this way, you ensure a clean separation between the build and runtime environments, which enhances security and maintainability.
Creating a .dockerignore
File
To reduce the size of your Docker image and improve build performance, exclude unnecessary files by creating a .dockerignore
file:
node_modules
npm-debug.log
dist
.next
.DS_Store
*.log
docker-compose.yml
This file acts like .gitignore
, preventing temporary files, logs, and other irrelevant directories from being copied into the Docker build context.
Building the Docker Image
With the Dockerfile in place, it’s time to build your Docker image. Open your terminal and run the following command:
docker build -t my-nextjs-app .
Here’s a detailed breakdown of the command:
- 🛠️
docker build
: The command used to build a Docker image from a Dockerfile. - 🏷️
-t my-nextjs-app
: Assigns a human-readable tag to the image (you can replacemy-nextjs-app
with any name you prefer). - 📂
.
: Specifies the current directory as the build context, where Docker will look for the Dockerfile and other necessary files.
The build process will output detailed logs, so watch for errors and ensure the image is created successfully.
Running the Docker Container
Once the image is built, you can create and run a container using the following command:
docker run -p 3000:3000 my-nextjs-app
Here’s what each flag and argument means:
- 🔌
-p 3000:3000
: Maps port 3000 on your host machine to port 3000 inside the container, allowing you to access the app viahttp://localhost:3000
. - 🐳
my-nextjs-app
: Specifies the name of the image to use for the container.
Visit the URL in your browser to confirm the app is running inside the container.
Using Docker Compose (Optional)
For more complex setups involving multiple services, Docker Compose simplifies the process. Create a docker-compose.yml
file:
version: "3.8"
services:
app:
build: .
ports:
- "3000:3000"
volumes:
- .:/app
- /app/node_modules
command: npm run dev
To start the app with Docker Compose, run:
docker-compose up
This command launches all services defined in the docker-compose.yml
file, making it an efficient way to manage multiple containers.
Deploying your containerized Next.js app
Once you've containerized your Next.js app and optimized it for production, it's time to deploy it to a hosting platform. Here are some popular options for deploying Docker containers:
Heroku
- Install the Heroku CLI and log in.
- Create a new Heroku app: heroku create
- Set the stack to container: heroku stack:set container
- Push your code: git push heroku main
DigitalOcean App Platform
- Create a new app on the DigitalOcean App Platform.
- Connect your GitHub repository.
- Configure your app to use the Dockerfile for deployment.
- Deploy your app.
AWS Elastic Beanstalk
- Create a new Elastic Beanstalk application.
- Choose the Docker platform.
- Upload your application code (including Dockerfile) as a zip file.
- Configure environment variables and other settings as needed.
- Deploy your application.
Google Cloud Run
- Build and push your Docker image to Google Container Registry.
- Create a new Cloud Run service.
- Select your container image and configure deployment options.
- Deploy your service.
Kubernetes
- Push your Docker image to a container registry.
- Create Kubernetes deployment and service YAML files.
- Apply the configurations to your Kubernetes cluster:
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
Best practices for Docker and Next.js
To make the most of Docker and Next.js in your development workflow, consider these best practices:
Use .dockerignore: Create a .dockerignore file to exclude unnecessary files from your Docker build context, improving build times and reducing image size.
Leverage caching: Structure your Dockerfile to take advantage of Docker's layer caching mechanism. Place commands that change less frequently (like installing dependencies) before commands that change more often (like copying application code).
Implement CI/CD: Set up continuous integration and deployment pipelines to automatically build, test, and deploy your containerized Next.js app.
Monitor performance: Use tools like New Relic, Datadog, or Prometheus to monitor the performance of your containerized application in production.
Implement secret management: Use environment variables or dedicated secret management tools to handle sensitive information, rather than hardcoding secrets in your Dockerfile or application code.
Regular updates: Keep your base images, Node.js version, and dependencies up to date to ensure you have the latest security patches and performance improvements.
Use Docker Compose for local development: Create a docker-compose.yml file to manage your development environment, including any necessary services like databases or caching layers.
Optimize for production: Use multi-stage builds and production-optimized Node.js settings to create smaller, more efficient containers for deployment.
Implement proper logging: Configure your Next.js app and Docker containers to output logs in a consistent, easily parseable format.
Practice security scanning: Regularly scan your Docker images for vulnerabilities using tools like Snyk, Clair, or Docker Scan.
Following these best practices, you'll create a more efficient, secure, and maintainable containerized Next.js application.
FAQ: about adding custom fonts to a Next.js project
Multi-stage builds help optimize the image size by separating the build process from the runtime environment, reducing unnecessary bloat.
Use the docker logs
command to view the container’s logs. For example: docker logs <container_id>
. You can also use docker exec
to open a shell inside the container for deeper debugging.
Update the dependencies in your package.json
file locally, rebuild the Docker image, and restart the container to apply the changes.
Yes, replace node:18
in the Dockerfile with the desired Node.js version or a custom base image.
Docker will stop at the failing step. Examine the logs to identify and fix the issue. You can also use Docker’s build caching features to streamline debugging.
Yes, Docker supports both static and server-rendered features of Next.js, including API routes. Ensure the server environment is correctly configured in the Dockerfile.
Use docker system prune
to remove unused images, containers, and volumes. Add the -a
flag to clean up all dangling objects.
Yes, you can deploy the container to any platform that supports Docker, such as AWS ECS, Google Kubernetes Engine, or Azure Container Instances.
Yes, push the image to a Docker registry like Docker Hub using docker push <username>/<image-name>
.
Use .dockerignore
, multi-stage builds, and minimal base images (e.g., node:alpine
) to create smaller, more efficient images.
Conclusion
Containerizing your Next.js application with Docker offers numerous benefits, from consistent development environments to simplified deployment processes. By following the steps and best practices outlined in this guide, you've learned how to create a Dockerfile, build and run Docker images, use Docker Compose for multi-container setups, optimize for production, and deploy your containerized app.
As you continue to work with Docker and Next.js, you'll discover even more ways to streamline your development workflow and improve your application's performance and scalability. Embrace the power of containerization, and watch your Next.js projects thrive in this modern development landscape.
Ready to take your Next.js development to the next level with Docker? Start containerizing your applications today and experience the benefits of consistent, scalable, and easily deployable web apps. If you found this guide helpful, share it with your fellow developers and join the conversation about containerization in web development. Have questions or want to share your experiences? Leave a comment below or reach out to our community forums for support and discussion. Image