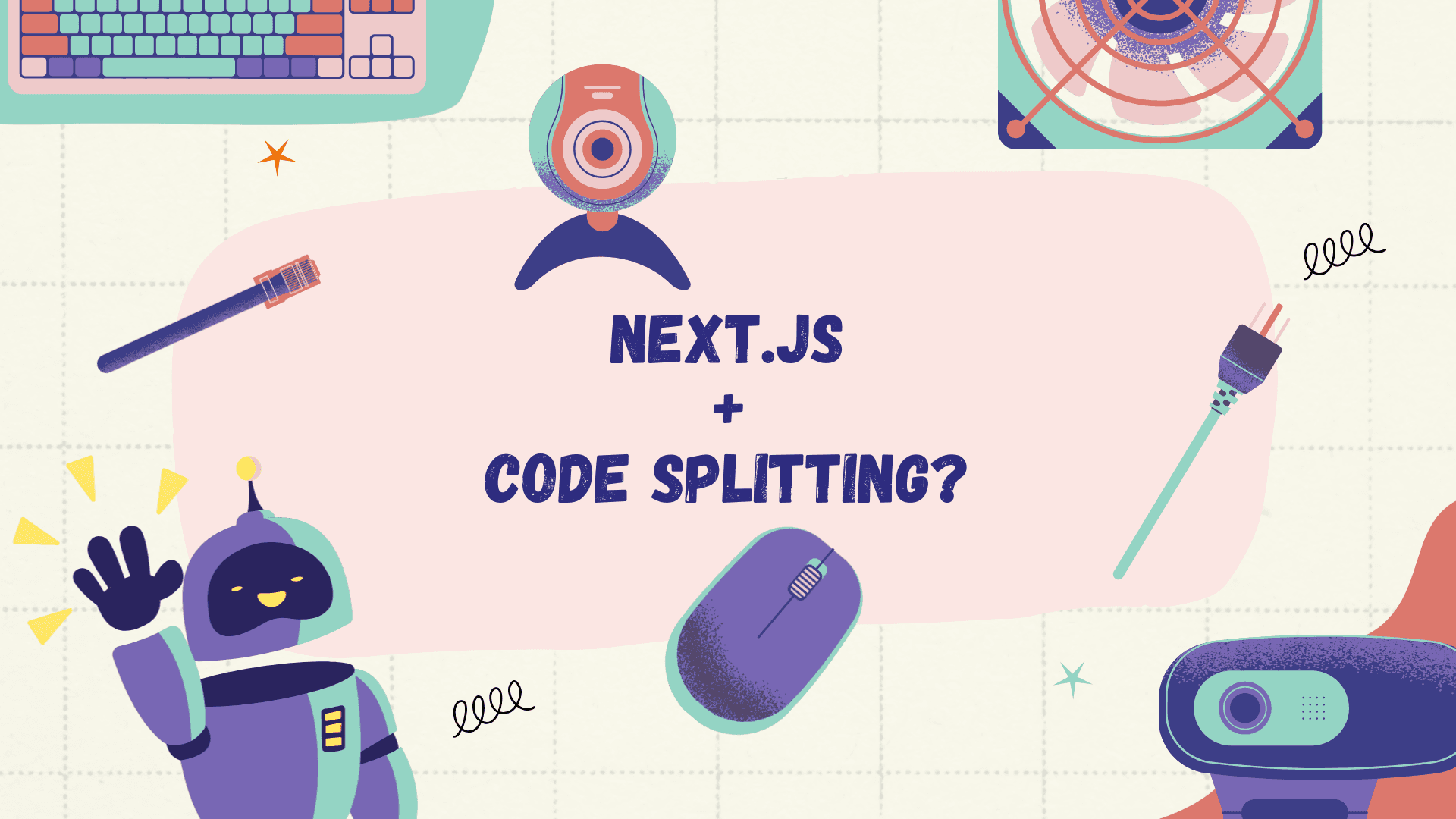
How does Next.js handle code splitting?
Next.js, a popular React framework, has gained widespread adoption for its ease of use, performance optimizations, and the seamless development experience it offers. One of its most significant features is automatic code splitting, which plays a crucial role in enhancing the performance of web applications by reducing the amount of code sent to the browser. This article delves into the mechanics of how Next.js handles code splitting, its benefits, and best practices for leveraging this feature to build fast, efficient applications.
Understanding Code Splitting
Code splitting is a technique that involves breaking down a web application's bundle into smaller, more manageable chunks. Instead of loading the entire application bundle upfront, code splitting allows you to load only the necessary code when it's needed. This results in faster page load times, improved user experience, and reduced bandwidth usage.
How Next.js Automates Code Splitting
Next.js takes an opinionated approach to code splitting, automating the process to ensure developers don't have to manually configure their applications to benefit from this optimization. Here's how it works:
Page-based Splitting
At its core, Next.js operates on a page-based routing system. Each page within the pages
directory gets its own JavaScript bundle. This means that when a user navigates to a specific page, only the code required for rendering that page is downloaded. This automatic per-page splitting ensures that the initial payload is as small as possible, speeding up the loading process.
Component-level Splitting with Dynamic Imports
Next.js also supports code splitting at the component level through dynamic imports. By using the dynamic()
function from Next.js, developers can specify components to be loaded lazily. This is particularly useful for heavy components that aren't immediately needed or for components that are only used under certain conditions. Dynamic imports allow these components to be fetched asynchronously when they're actually required, further optimizing the application's performance.
Vendor Chunking
Common libraries and frameworks used across different parts of an application can significantly increase the size of JavaScript bundles. Next.js intelligently identifies common modules used across multiple pages and extracts them into a separate "vendor" chunk. This ensures that libraries and frameworks are loaded once and cached by the browser, reducing the amount of code that needs to be downloaded as users navigate through the application.
Webpack Under the Hood
Next.js leverages Webpack, a powerful module bundler, to implement its code splitting strategy. Webpack's dynamic imports, chunking capabilities, and tree-shaking (removing unused code) are utilized by Next.js to efficiently split code. The framework wraps these features in a developer-friendly abstraction, eliminating the need for manual configuration.
Benefits of Next.js Code Splitting
- Faster Page Loads: By loading only the necessary code, applications boot up more quickly, enhancing the user experience.
- Reduced Bandwidth Usage: Smaller initial payloads mean less data consumption, which is particularly beneficial for users on limited or slow internet connections.
- Improved SEO: Faster loading times contribute to better search engine rankings, as page speed is a known ranking factor.
Best Practices for Maximizing Code Splitting Benefits
- Use Dynamic Imports Judiciously: While dynamic imports are powerful, overusing them can lead to excessive network requests. Balance their use based on the size and necessity of components.
- Analyze Bundle Size: Regularly use Next.js's built-in analyzing tools or Webpack bundle analyzers to identify and address code bloat.
- Prefetch Important Resources: Next.js allows prefetching pages and assets. Use this feature to preload important resources that the user is likely to interact with next.
Advanced Insights into Next.js Code Splitting
Building on the foundational understanding of how Next.js handles code splitting, let's delve deeper into the mechanics, optimizations, and nuanced strategies that Next.js employs to further refine and enhance application performance.
Optimizing Dynamic Imports
Dynamic imports in Next.js not only allow for component-level code splitting but also support prefetching. This means that while the initial page load only requests the necessary chunks, Next.js can prefetch additional chunks in the background, preparing for subsequent navigation actions. Developers can control this prefetching behavior through the next/link
or next/router
modules, specifying which components or pages should be preloaded to ensure instant transitions.
Server-Side Rendering (SSR) and Code Splitting
Next.js's code splitting works seamlessly with its server-side rendering capabilities. When a page is rendered on the server, Next.js ensures that only the necessary chunks are included in the response sent to the browser. This SSR-code splitting integration optimizes the time to first byte (TTFB), ensuring that users perceive fast page loads, while the rest of the application's code is loaded in the background or on-demand.
Static Generation and Automatic Static Optimization
Next.js's automatic static optimization feature can further enhance the benefits of code splitting. Pages without dynamic data dependencies are pre-rendered to static HTML at build time. Combined with code splitting, this means that the browser loads minimal JavaScript to become interactive, significantly improving performance metrics like First Contentful Paint (FCP) and Time to Interactive (TTI).
Customizing Babel and Webpack Configurations
Although Next.js abstracts away much of the configuration complexity, it still offers hooks for customizing the underlying Babel and Webpack configurations. This is crucial for advanced optimizations where the default code splitting strategy might need adjustments. For instance, developers can modify the Webpack configuration to change chunking behavior, such as creating more granular chunks, adjusting the size thresholds for splitting, or customizing the naming conventions for easier analysis.
Analyzing and Optimizing Bundle Size
Next.js integrates well with tools like webpack-bundle-analyzer
to provide insights into the bundle structure and size. Regularly analyzing the bundle can uncover opportunities for optimization, such as identifying large dependencies that could be replaced with lighter alternatives, or spotting duplicated code across chunks that could be better shared. This proactive bundle management is key to maintaining fast, efficient applications as they scale.
Lazy Loading Images and Assets
Beyond JavaScript code, Next.js also optimizes the loading of images and other assets. The next/image
component supports automatic image optimization, resizing, and lazy loading, ensuring that images only load when they enter the viewport. This strategy complements code splitting by reducing initial page weight and load times, further enhancing the user experience.
The Impact of Incremental Static Regeneration (ISR)
Incremental Static Regeneration (ISR) offers a middle ground between full static generation and server-side rendering, allowing pages to be updated in the background after being served. When combined with code splitting, ISR ensures that users always receive fast, up-to-date content without unnecessary loading delays, making it an excellent choice for dynamic content that doesn't change on every request.
Conclusion
Next.js's approach to code splitting is a sophisticated blend of automatic optimizations and developer-controlled strategies. By understanding and leveraging these mechanisms, developers can significantly improve the performance and user experience of their web applications. Whether through smart chunking, prefetching, or integration with Next.js's rendering modes, there's a wealth of opportunities to optimize loading times and resource usage. As web applications continue to grow in complexity, the importance of efficient code splitting and bundle optimization cannot be overstated. Next.js provides the tools and abstractions necessary to achieve these goals with minimal overhead, allowing developers to focus on building feature-rich, performant applications.