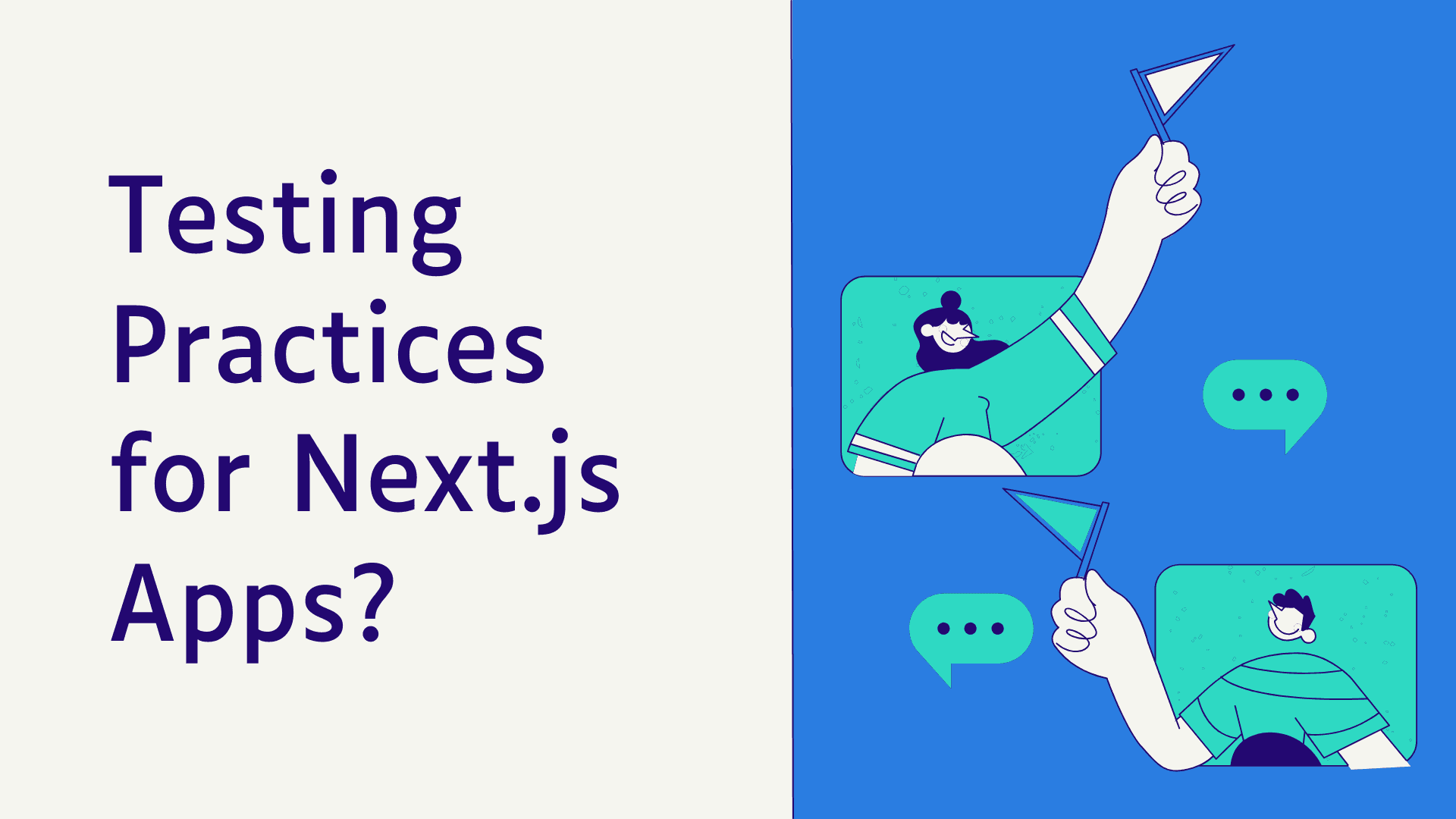
What are the recommended testing practices for Next.js apps?
Testing is an essential aspect of software development, providing assurance of code quality and functionality. In the context of Next.js, a popular React framework known for its server-side rendering and static generation features, testing practices need to be tailored to its unique architecture. This article discusses recommended testing practices for Next.js applications, covering various levels of testing and essential tools.
Understanding the Testing Pyramid in Next.js
The testing pyramid is a concept that illustrates the ideal balance of testing types in a software project. In Next.js applications, this typically involves:
- Unit Testing: Testing individual components or functions in isolation.
- Integration Testing: Testing the interaction between different parts of the application.
- End-to-End (E2E) Testing: Testing the entire application flow as it would occur in a real user environment.
Unit Testing in Next.js
Unit tests are the foundation of a solid testing strategy. They are quick to run and help catch bugs early.
Tools
- Jest: A popular testing framework that works well with Next.js for running tests and providing assertions.
- React Testing Library: Aims to test components in a way that resembles how users interact with them.
Practices
- Isolate Components: Test React components in isolation. Mock external dependencies using Jest's mocking capabilities.
- Test Functionality, Not Implementation: Focus on what the component does, not how it does it. Avoid testing internal state or lifecycle methods directly.
- Code Coverage: Strive for high test coverage but prioritize meaningful tests over simply hitting coverage metrics.
Integration Testing
Integration testing in Next.js involves testing how different components and services work together.
Tool
- Jest along with React Testing Library can be used for integration tests as well.
- MSW (Mock Service Worker): Useful for mocking API responses in a realistic manner.
Practice
- Test Component Integration: Verify that components work correctly when used together.
- Mock External Services: Use MSW to mock API calls and test the interaction between your application and external services.
- Routing and Data Fetching: Test scenarios involving Next.js's
getServerSideProps
orgetStaticProps
.
End-to-End (E2E) Testing
E2E testing checks the application's flow from start to finish. It's crucial for ensuring the user experience is as expected.
Key Tools
- Cypress or Playwright: Powerful tools for writing E2E tests that mimic real-user interactions.
- Puppeteer: Another option, especially useful for testing server-side rendered pages.
Key Practices
- Real User Flows: Focus on critical paths that a user is most likely to take.
- Responsive and Cross-Browser Testing: Ensure your application works across different devices and browsers.
- CI/CD Integration: Automate E2E tests in your Continuous Integration/Continuous Deployment pipeline.
Additional Testing Considerations
- Visual Regression Testing: Tools like Percy or Chromatic can be integrated to detect UI changes that might not be caught by other types of tests.
- Accessibility Testing: Incorporate tools like axe-core or pa11y to ensure your application is accessible.
- Performance Testing: Use Lighthouse or similar tools to test and monitor the performance of your Next.js application.
Continuing with our discussion on testing practices for Next.js applications, let's delve deeper into strategies and considerations that can enhance the quality and effectiveness of your testing efforts.
Advanced Unit Testing Techniques
While we've covered the basics of unit testing, there are additional strategies that can further improve the quality of your tests:
Component Edge Cases
- Props Variation: Test how components behave with different props, including edge cases and error conditions.
- Event Handling: Ensure that events are correctly handled and trigger the expected outcomes.
Mocking Strategies
- Deep Mocking: For complex dependencies, consider deep mocking to simulate intricate behaviors.
- Manual Mocks: For modules specific to Next.js (like 'next/router'), create manual mocks that replicate the module's behavior in a controlled testing environment.
Enhanced Integration Testing Approaches
Integration tests in a Next.js context often require a careful balance between unit testing principles and a broader scope that includes multiple components and their interactions.
Page-Level Testing
- Page Components: Test entire pages as a unit, ensuring that the integration of various components on the page works as intended.
- API Route Testing: Test the API routes provided by Next.js, which can include server-side logic and database interactions.
Global State Management
- If your application uses global state management (like Redux or Context API), test how components interact with global state under different scenarios.
Optimizing E2E Tests
E2E tests are the most resource-intensive, so optimizing them is crucial for maintaining an efficient testing pipeline.
Selective Testing
- Critical Path Focus: Prioritize E2E tests for critical user paths. Not every feature needs an E2E test.
- Smoke Tests: Implement a suite of smoke tests that run on every deployment to quickly catch major issues.
Advanced Techniques
- Visual Comparisons: Incorporate screenshot testing within E2E tests to catch visual regressions.
- Network Stubbing: Use network stubbing to simulate server responses, testing how the application behaves under different server-side conditions.
Performance and Load Testing
Performance testing is another critical aspect, especially for a server-side rendering framework like Next.js.
Tools and Techniques
- Load Testing: Tools like k6 or Apache JMeter can simulate high traffic and analyze how your application performs under load.
- Server-Side Rendering Performance: Test the performance of your server-side rendering, focusing on TTFB (Time to First Byte) and overall page load times.
Continuous Integration and Deployment
Incorporating testing into your CI/CD pipeline ensures that tests are run automatically, leading to early detection of issues.
CI/CD Practices
- Automate Testing: Configure your CI/CD system to run unit, integration, and selected E2E tests on every push or pull request.
- Quality Gates: Set up quality gates that prevent code from being merged if tests fail or if code coverage falls below a certain threshold.
Testing in a DevOps Culture
- Shift-Left Testing: Encourage testing early in the development cycle. This includes involving QA in planning and review stages and enabling developers to write and run tests locally.
- Monitoring and Observability: Post-deployment, use monitoring and observability tools to track application performance and user behavior, feeding insights back into the testing process.
Adopting a comprehensive and well-thought-out testing strategy for Next.js applications not only helps in delivering a robust product but also fosters a culture of quality within the development team. By combining various testing types and practices, and integrating them into the development and deployment workflows, teams can ensure that their Next.js applications meet the highest standards of functionality, performance, and user experience. Remember, effective testing is not a one-time task but an ongoing process that evolves with your application.
Conclusion
In summary, effective testing in Next.js applications involves a combination of unit, integration, and E2E tests. By leveraging tools like Jest, React Testing Library, MSW, and Cypress, and following best practices tailored to Next.js's features, developers can ensure robust, reliable, and user-friendly applications. Remember, the goal of testing is not just to find bugs, but to create a culture of quality and prevent issues from arising in the first place.