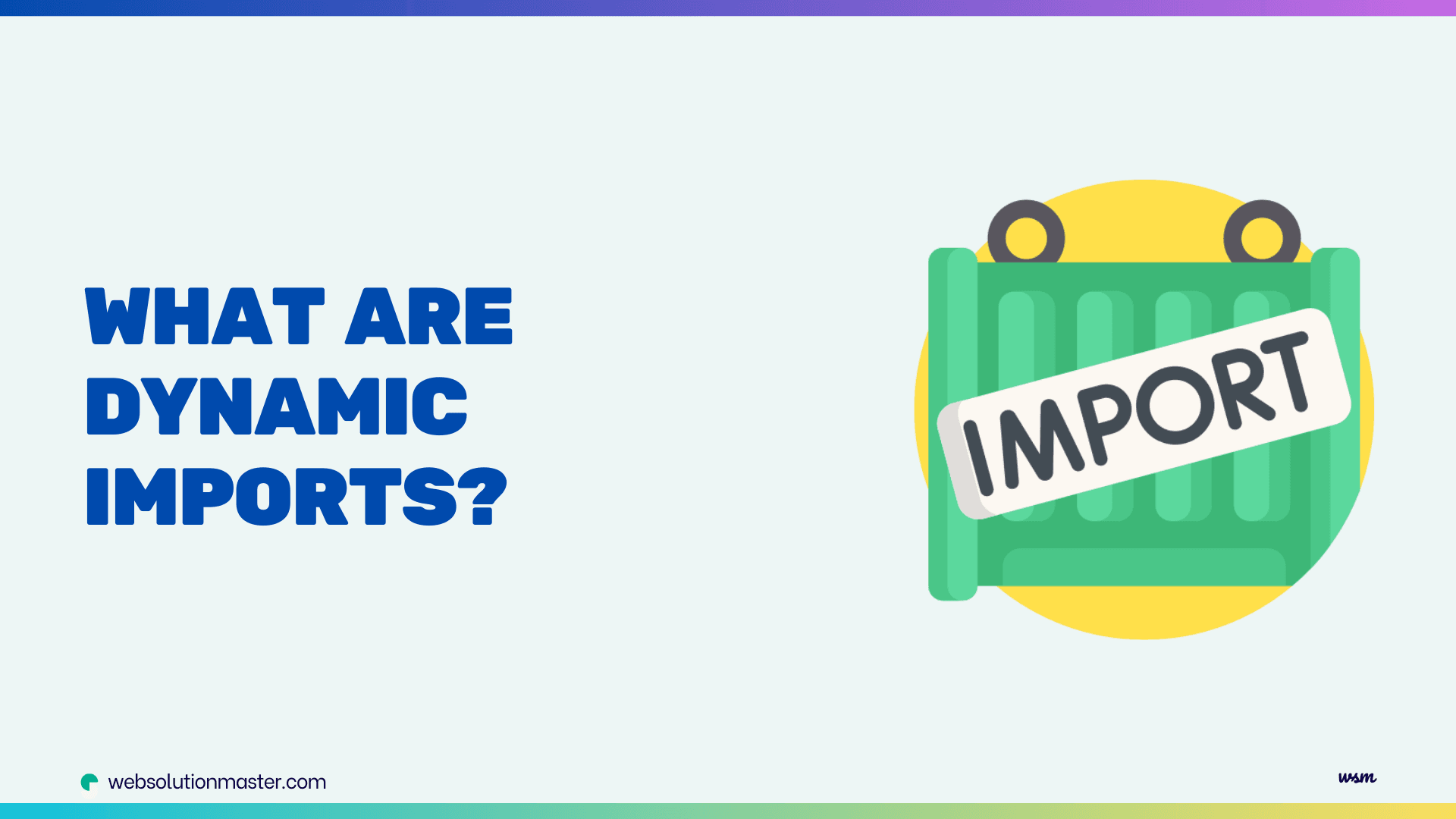
What are dynamic imports & how are they used in Next.js?
Next.js, the React framework for server-side rendering and static site generation, has revolutionized the way web applications are built. One of its powerful features is dynamic imports, which allows you to load code on-demand, enhancing performance and optimizing resource utilization. In this article, we'll delve into the intricacies of dynamic imports and how they can supercharge your Next.js applications.
Dynamic imports are a powerful feature in JavaScript, particularly within the Next.js framework, that allows developers to load modules on demand rather than at the initial load time. This can significantly improve the performance and user experience of web applications by reducing the initial bundle size and deferring the loading of non-essential code.
In this article, we'll delve into what dynamic imports are, their benefits, and how to use them effectively in Next.js.
What are Dynamic Imports?
Dynamic imports enable the importation of JavaScript modules asynchronously. Unlike static imports, which are processed at compile time and included in the initial bundle, dynamic imports are resolved at runtime. This means the module is fetched and executed only when needed.
The syntax for a dynamic import in JavaScript looks like this:
import("./module").then((module) => {
// Use the module here
});
This feature is particularly useful for splitting code and loading modules on demand, which can lead to performance optimizations.
Understanding the concept of code splitting
Before diving into dynamic imports, it's essential to grasp the concept of code splitting. In traditional web development, all JavaScript files are bundled together, resulting in a single, monolithic file. While this approach simplifies deployment, it can lead to slow load times, especially for larger applications. Code splitting addresses this issue by dividing the codebase into smaller chunks, allowing browsers to load only the necessary code initially and fetch additional chunks as needed.
Benefits of dynamic imports in Next.js
Dynamic imports offer several benefits that make them a game-changer for web development:
Improved Performance: By loading code on-demand, dynamic imports reduce the initial bundle size, leading to faster page load times and improved user experience.
Efficient Resource Utilization: Since only the required code is loaded, dynamic imports optimize resource usage, conserving bandwidth and minimizing memory footprint.
Lazy Loading: Dynamic imports enable lazy loading, where components or modules are loaded only when they are needed, further enhancing performance.
Modular Development: With dynamic imports, you can structure your application into modular components, promoting code reusability and maintainability.
Conditional Loading: Modules can be loaded based on user actions or conditions, ensuring that only the necessary code is loaded and executed.
Improved User Experience: By deferring the loading of non-critical parts of your application, users can start interacting with your app sooner, improving perceived performance.
Reduced Bundle Size: Dynamic imports help in keeping the initial JavaScript bundle small, which is crucial for improving load times and reducing bandwidth usage, especially on slow networks.
Performance Optimization: By splitting code into smaller chunks and loading them as needed, the initial load time of your application is reduced. This results in a faster initial rendering and a more responsive user experience.
Using Dynamic Imports in Next.js
Next.js provides a straightforward way to leverage dynamic imports. Instead of using the traditional import statement, you can use the dynamic import() syntax, which returns a Promise that resolves with the requested module.
Next.js, a popular React framework, provides robust support for dynamic imports out of the box. Here's how you can leverage dynamic imports in a Next.js application:
Basic Usage
To dynamically import a module in a Next.js project, you can use the next/dynamic
function. Here's a simple example:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(() => import("./components/DynamicComponent"));
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
In this example, the DynamicComponent
is only loaded when it is needed, rather than at the initial page load.
With Loading Component
Sometimes, you might want to display a loading indicator while the dynamic component is being loaded. You can achieve this by passing a loading
component to dynamic
:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading...</p>,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Prefetching Dynamic Components
Next.js supports prefetching for dynamic components, which can improve performance by preloading components before they are needed. This can be enabled by setting the ssr
option to false:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
ssr: false,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Disabling Server-Side Rendering
In some cases, you may want to disable server-side rendering for a dynamic component. This can be useful for components that rely on browser-specific APIs or features that are not available on the server:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
ssr: false,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Custom Error Handling
You can also handle errors that occur during the dynamic import by passing an onError
function:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading...</p>,
ssr: false,
onError: (err) => {
console.error("Failed to load the component", err);
},
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Example with React Suspense
Next.js also supports React's Suspense
for dynamic imports, providing a more declarative way to handle loading states:
import React, { Suspense } from "react";
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
suspense: true,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<Suspense fallback={<div>Loading...</div>}>
<DynamicComponent />
</Suspense>
</div>
);
export default HomePage;
Certainly! Let's dive deeper into the advanced usage scenarios, best practices, and common pitfalls associated with dynamic imports in Next.js.
Advanced Usage Scenarios
Dynamic imports are not just limited to components; they can also be used for libraries, utilities, and more complex configurations.
Dynamic Imports for Libraries
Sometimes, large third-party libraries are not immediately needed. You can dynamically import these libraries to optimize performance:
import dynamic from "next/dynamic";
const SomeLargeLibrary = dynamic(() => import("some-large-library"), {
ssr: false,
loading: () => <p>Loading library...</p>,
});
const HomePage = () => {
const handleClick = async () => {
const lib = await import("some-large-library");
// Use the library as needed
};
return (
<div>
<h1>Welcome to Next.js!</h1>
<button onClick={handleClick}>Load Library</button>
</div>
);
};
export default HomePage;
Dynamic Import with Hooks
Dynamic imports can be effectively used with React hooks to load components or modules based on user interactions:
import { useState, useEffect } from "react";
import dynamic from "next/dynamic";
const HomePage = () => {
const [DynamicComponent, setDynamicComponent] = useState(null);
useEffect(() => {
const loadComponent = async () => {
const component = await import("./components/DynamicComponent");
setDynamicComponent(component.default);
};
loadComponent();
}, []);
return (
<div>
<h1>Welcome to Next.js!</h1>
{DynamicComponent ? <DynamicComponent /> : <p>Loading...</p>}
</div>
);
};
export default HomePage;
Conditional Dynamic Imports
Load components based on specific conditions, such as user roles or feature flags:
import dynamic from "next/dynamic";
import { useEffect, useState } from "react";
const AdminComponent = dynamic(() => import("./components/AdminComponent"), {
loading: () => <p>Loading...</p>,
});
const UserComponent = dynamic(() => import("./components/UserComponent"), {
loading: () => <p>Loading...</p>,
});
const HomePage = ({ userRole }) => {
const [Component, setComponent] = useState(null);
useEffect(() => {
if (userRole === "admin") {
setComponent(() => AdminComponent);
} else {
setComponent(() => UserComponent);
}
}, [userRole]);
return (
<div>
<h1>Welcome to Next.js!</h1>
{Component && <Component />}
</div>
);
};
export default HomePage;
Performance improvements with dynamic imports
Dynamic imports can significantly enhance the performance of your Next.js applications by reducing the initial bundle size and optimizing resource utilization. Here's how:
-
Reduced Initial Bundle Size: By loading only the essential code initially, dynamic imports minimize the initial bundle size, resulting in faster page load times.
-
Optimized Resource Utilization: Since only the required code is loaded, dynamic imports optimize resource usage, conserving bandwidth and minimizing memory footprint.
-
Improved User Experience: Faster page load times and smoother transitions between components create a better user experience, leading to increased engagement and satisfaction.
Real-world examples of dynamic imports in Next.js
Dynamic imports can be applied in various scenarios within Next.js applications. Here are a few real-world examples:
-
Lazy Loading Components: Lazy load non-critical components or those used infrequently, such as modals, tooltips, or complex visualizations.
-
Feature-based Code Splitting: Split your application's features into separate chunks, allowing users to download only the necessary code for their current context.
-
Third-party Library Integration: Dynamically import third-party libraries or dependencies to reduce the initial bundle size and improve load times.
-
Internationalization (i18n): Load language-specific translations or resources on-demand, optimizing performance for multilingual applications.
Best practices for using dynamic imports in Next.js
While dynamic imports offer numerous benefits, it's essential to follow best practices to ensure optimal performance and maintainability:
-
Avoid Excessive Imports: Dynamically importing too many modules can lead to code fragmentation and potential performance issues. Strike a balance between code splitting and maintainability.
-
Preload Critical Components: Preload essential components or modules that are required on initial page load to avoid delays and improve perceived performance.
-
Leverage Code Splitting Utilities: Next.js provides built-in utilities like next/dynamic and next/loadable to simplify dynamic imports and code splitting.
-
Monitor Bundle Size: Regularly monitor your application's bundle size and optimize accordingly to ensure optimal performance.
-
Test and Optimize: Thoroughly test your application's performance with dynamic imports and optimize as needed, considering factors like network conditions and device capabilities.
Only Load When Necessary
Avoid unnecessary dynamic imports. Only use dynamic imports for code that is genuinely optional or secondary in importance to the initial user experience.
Use Loading Indicators
Always provide feedback to users when dynamically loading components. A simple loading spinner or message improves the user experience.
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading component...</p>,
},
);
Prefetch Critical Components
For components that are critical but can be deferred slightly, use prefetching to load them just before they are needed:
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
ssr: false,
loading: () => <p>Loading...</p>,
},
);
Error Handling
Implement error handling to manage scenarios where the dynamic import fails. This prevents the application from crashing and can provide useful feedback to the user.
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading...</p>,
ssr: false,
onError: (error) => {
console.error("Error loading component:", error);
},
},
);
Potential pitfalls and how to avoid them
While dynamic imports offer numerous benefits, there are potential pitfalls to be aware of:
Increased Complexity: Dynamic imports can introduce complexity in code organization and management, especially in larger applications. Maintain a clear structure and documentation to mitigate this issue.
Redundant Downloads: If not implemented correctly, dynamic imports can lead to redundant downloads of the same module, negating the performance benefits. Proper caching and bundling strategies can help avoid this pitfall.
Synchronization Issues: When working with dynamic imports, synchronization issues can arise, such as components rendering before their dependencies are loaded. Proper error handling and loading state management are crucial.
Server-side Rendering (SSR) Challenges: Dynamic imports can complicate server-side rendering, as the imported modules need to be available on both the server and client. Next.js provides utilities like next/dynamic to simplify this process.
SEO Considerations
Dynamic imports can impact SEO because search engines may not execute JavaScript to load all dynamic components. Ensure critical content is statically rendered or use server-side rendering (SSR) where necessary.
Performance Overhead
While dynamic imports can reduce the initial load time, overusing them or incorrectly configuring them can lead to performance overhead. Balance between static and dynamic imports to optimize both initial load and runtime performance.
Maintaining State
Be cautious with state management when using dynamic imports. Ensure that the state is properly managed and passed to dynamically imported components to avoid unexpected behavior.
Comparison with traditional import statements
Traditional import statements load all the required modules upfront, resulting in a larger initial bundle size. While this approach simplifies code organization, it can lead to slower page load times and inefficient resource utilization, especially for larger applications.
Dynamic imports, on the other hand, allow for on-demand code loading, reducing the initial bundle size and optimizing resource usage. However, they introduce additional complexity in code organization and management, requiring careful planning and implementation.
The choice between traditional imports and dynamic imports depends on the specific requirements of your application, such as performance goals, codebase size, and development team preferences.
Future developments and enhancements for dynamic imports in Next.js
The Next.js team is continuously working on improving dynamic imports and code splitting capabilities. Some potential future developments and enhancements include:
Improved Tooling: Enhanced tooling and utilities to simplify dynamic import management, bundling, and optimization.
Advanced Code Splitting Strategies: More advanced code splitting strategies, such as route-based code splitting or component-level code splitting, to further optimize performance.
Automatic Code Splitting: Automatic code splitting based on predefined rules or heuristics, reducing the need for manual intervention.
Integration with Web Bundlers: Tighter integration with popular web bundlers like Webpack or Rollup, enabling more advanced code splitting and optimization techniques.
Performance Monitoring and Analytics: Built-in performance monitoring and analytics tools to help identify and optimize potential performance bottlenecks related to dynamic imports.
Dynamic Imports in Next.js: FAQ
Dynamic imports in Next.js allow you to import JavaScript modules asynchronously. Instead of including all code in the initial bundle, dynamic imports enable code to be loaded on demand. This can improve the performance and user experience by reducing the initial load time and deferring the loading of non-critical code.
To use dynamic imports in Next.js, you can use the next/dynamic
function. Here’s a basic example:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(() => import("./components/DynamicComponent"));
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
- Performance Optimization: Reduces the initial bundle size and load time.
- Improved User Experience: Loads only the necessary code when needed.
- Conditional Loading: Modules can be loaded based on user actions or specific conditions.
- Reduced Bandwidth Usage: Less initial code to download means faster loading times on slower networks.
Yes, you can provide a loading component while the dynamic import is being fetched. Here’s an example:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading...</p>,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
You can handle errors by using the onError
callback in the dynamic
function:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
loading: () => <p>Loading...</p>,
ssr: false,
onError: (err) => {
console.error("Failed to load the component", err);
},
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Yes, you can use dynamic imports with React hooks. Here’s an example:
import { useState, useEffect } from "react";
import dynamic from "next/dynamic";
const HomePage = () => {
const [DynamicComponent, setDynamicComponent] = useState(null);
useEffect(() => {
const loadComponent = async () => {
const component = await import("./components/DynamicComponent");
setDynamicComponent(component.default);
};
loadComponent();
}, []);
return (
<div>
<h1>Welcome to Next.js!</h1>
{DynamicComponent ? <DynamicComponent /> : <p>Loading...</p>}
</div>
);
};
export default HomePage;
Next.js can prefetch dynamically imported components to improve performance. Prefetching can be enabled by setting the ssr
option to false
:
import dynamic from "next/dynamic";
const DynamicComponent = dynamic(
() => import("./components/DynamicComponent"),
{
ssr: false,
},
);
const HomePage = () => (
<div>
<h1>Welcome to Next.js!</h1>
<DynamicComponent />
</div>
);
export default HomePage;
Dynamic imports can impact SEO since search engines may not execute JavaScript to load all dynamic components. To ensure critical content is rendered for SEO purposes, use server-side rendering (SSR) for essential components.
Avoid using dynamic imports when:
- The module is critical for the initial page load.
- The module is small and would not significantly impact the bundle size.
- The module needs to be SEO-friendly and should be rendered on the server.
Dynamic imports and lazy loading are similar in that they both aim to load code on demand. However, dynamic imports specifically refer to the JavaScript feature of importing modules asynchronously using the import()
syntax, whereas lazy loading is a broader concept that can apply to images, components, or other resources.
Yes, dynamic imports can be used for libraries or utilities. This can be especially useful for large libraries that are not immediately needed:
const handleClick = async () => {
const { someFunction } = await import("some-large-library");
someFunction();
};
Dynamic imports are a versatile feature that can help you optimize the performance and user experience of your Next.js applications. By understanding and leveraging dynamic imports effectively, you can create more efficient and responsive web applications.
Conclusion
Dynamic imports in Next.js are a game-changer for web development, offering significant performance improvements and efficient resource utilization. By loading code on-demand, dynamic imports reduce the initial bundle size, enhance page load times, and optimize resource usage, creating a better user experience.
While dynamic imports introduce some complexity, following best practices and leveraging Next.js utilities can help mitigate potential pitfalls. As the Next.js ecosystem continues to evolve, we can expect even more powerful tools and features for dynamic imports and code splitting, further solidifying Next.js as a leading framework for building modern, performant web applications. Unlock the full potential of your Next.js applications with dynamic imports. Reach out to our team of experienced Next.js developers to learn how we can help you implement this powerful feature and optimize your web application's performance. Contact us today for a free consultation and take the first step towards building lightning-fast, resource-efficient web experiences that delight your users.
Key Takeaways
- Performance Optimization: Use dynamic imports to load non-essential code on demand, reducing initial load time.
- User Experience: Provide loading indicators and handle errors gracefully to maintain a smooth user experience.
- Balance and Strategy: Strategically decide which parts of your application to load dynamically to achieve the best performance.
Dynamic imports are a versatile tool in your development toolkit. With thoughtful implementation, they can significantly contribute to building high-performing, responsive, and user-friendly Next.js applications.