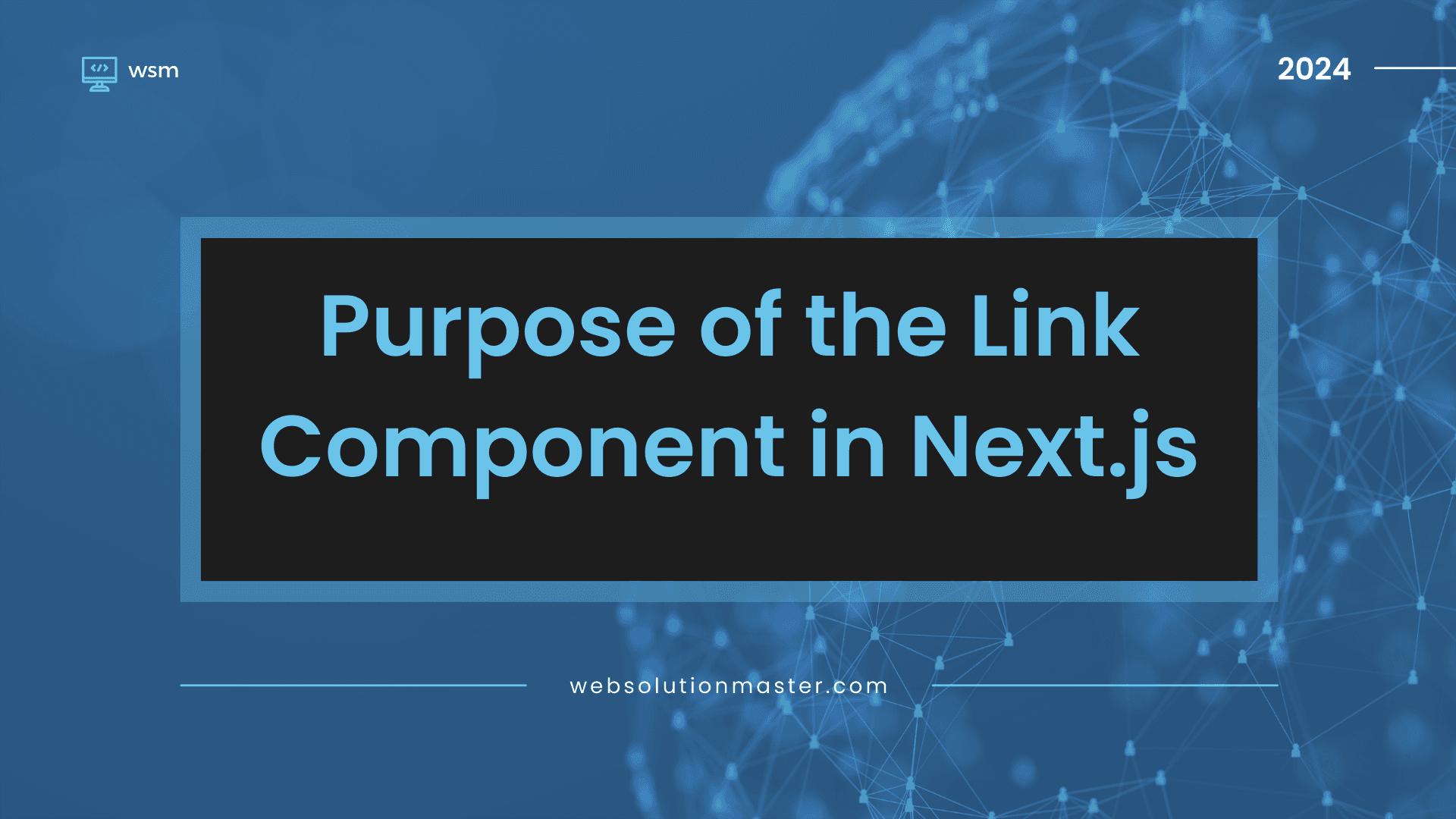
Purpose of the Link Component in Next.js
Next.js, a popular framework for building React applications, is designed to provide developers with the tools they need to create fast and user-friendly web applications. Among its many features, the Link
component stands out as a critical element for enabling efficient navigation within Next.js projects. This article explores the purpose of the Link
component in Next.js, its benefits, and how it contributes to the development of modern web applications.
Introduction to Next.js and Client-Side Navigation
Before delving into the specifics of the Link
component, it's essential to understand the context in which Next.js operates. Next.js is built on top of React, extending its capabilities to include server-side rendering, static site generation, and more, all aimed at improving performance and enhancing the user experience. A fundamental part of creating a seamless web application is enabling users to navigate between different pages without the need for a full page reload, which is where client-side navigation comes into play.
Client-side navigation refers to the process of changing views within a web application without making a new request to the server to fetch a new page. This approach significantly improves the user experience by reducing load times and creating a more dynamic and responsive application.
The Role of the Link Component
The Link
component in Next.js is specifically designed to facilitate client-side navigation. It allows developers to create hyperlinks that users can interact with to navigate between different parts of an application without the traditional round-trip to the server. Here's a closer look at the purpose and benefits of using the Link
component:
Enhanced Performance
By avoiding full page reloads, the Link
component contributes to faster navigation between pages. This speed is crucial for maintaining a smooth user experience, particularly in dynamic applications where users expect quick interactions.
Simplified Routing
Next.js provides a file-based routing system, where the file structure in the pages
directory corresponds to the application's routes. The Link
component works seamlessly with this system, making it straightforward to link to different pages by specifying the path to the target page in the href
attribute.
Prefetching Capabilities
One of the unique features of the Link
component is its ability to prefetch pages. Next.js can automatically prefetch the code for the linked page when the link comes into the viewport or when the mouse hovers over the link. This means that the page is ready to be rendered instantly when the user clicks the link, further enhancing the navigation speed.
Seamless Integration with Dynamic Routes
Next.js supports dynamic routing, allowing developers to create pages that can match a variety of paths. The Link
component integrates smoothly with dynamic routes, enabling developers to pass parameters through the URL and navigate to pages that require dynamic data fetching based on those parameters.
Customizable with a Familiar API
While the Link
component is powerful, it remains simple and customizable. It acts as a wrapper around the anchor (<a>
) tag, meaning developers can still use familiar attributes like className
for styling. However, it's important to note that the href
attribute is passed to the Link
component, and the actual anchor tag inside it can be styled or receive other attributes like title
or id
.
Using the Link Component
Implementing the Link
component in a Next.js application is straightforward. Here's a basic example:
import Link from "next/link";
const Navigation = () => (
<nav>
<Link href="/about">
<a>About Us</a>
</Link>
<Link href="/contact">
<a>Contact</a>
</Link>
</nav>
);
In this example, the Link
component is used to create navigational links to the /about
and /contact
pages. Notice how the href
attribute specifies the destination, and the anchor tag is used as a child of Link
to provide the clickable element.
Advanced Usage and Best Practices with the Link Component
While the basics of the Link
component cover most needs, understanding its advanced usage and adhering to best practices can significantly enhance your Next.js applications. Let’s delve into some of these aspects to maximize the effectiveness of client-side navigation.
Dynamic Linking and Path Parameters
Next.js's dynamic routes allow for the creation of pages that adapt based on the URL parameters. The Link
component handles these dynamic routes gracefully. When linking to a dynamic route, you can pass an object to the href
attribute, specifying the pathname along with the query parameters:
import Link from "next/link";
const PostLink = ({ postId }) => (
<Link href={{ pathname: "/post/[pid]", query: { pid: postId } }}>
<a>Read Post</a>
</Link>
);
In this example, we're linking to a dynamic route (/post/[pid]
) and providing the pid
parameter via the query object. This pattern is particularly useful when you need to generate links based on data fetched from an API or a database.
Prefetching Control
While automatic prefetching is a powerful feature for enhancing performance, there might be scenarios where you want to control this behavior. For instance, if you have a large number of links visible on the screen, prefetching all of them might not be efficient. The Link
component allows you to disable prefetching by setting the prefetch
prop to false
:
<Link href="/about" prefetch={false}>
<a>About Us</a>
</Link>
Leveraging the Replace Prop
The Link
component also supports a replace
prop, which, when set to true, replaces the current history state instead of adding a new one. This is akin to calling replaceState
instead of pushState
on the window.history
API. This option can be useful in situations where you do not want the user to go back to the previous page in the history stack:
<Link href="/home" replace>
<a>Go to Home</a>
</Link>
Styling Links
While the Link
component itself does not accept style attributes (since it does not render any visible HTML), you can apply styles directly to the child <a>
tag or use CSS classes. For conditional styling based on the current route, consider using the useRouter
hook from Next.js to add active class styles:
import { useRouter } from "next/router";
const NavLink = ({ href, children }) => {
const router = useRouter();
const isActive = router.pathname === href;
return (
<Link href={href}>
<a style={{ color: isActive ? "red" : "blue" }}>{children}</a>
</Link>
);
};
Best Practices
- Use the
<Link>
Component for Internal Navigation: For navigating within your Next.js application, always use theLink
component to take advantage of its client-side navigation capabilities. For external links, use the standard<a>
tag with anhref
attribute. - Avoid Unnecessary Prefetching: While prefetching can improve performance, overusing it, especially on pages with many links, can lead to excessive data usage and bandwidth consumption. Use prefetching judiciously.
- Accessibility: Ensure your links are accessible. This means providing meaningful text within the
<a>
tag for screen readers and setting thetitle
attribute when necessary to offer additional context.
Conclusion
The Link
component is a fundamental building block for creating dynamic and efficient web applications with Next.js. By understanding and utilizing its full capabilities, developers can craft applications that not only load quickly but also provide a fluid and engaging user experience. Remember to follow best practices and consider the broader implications of features like prefetching and dynamic routing to build robust Next.js applications.
The Link
component is a cornerstone of Next.js, empowering developers to build applications that are not only fast and efficient but also provide a superior user experience through seamless client-side navigation. By leveraging the Link
component's features, such as prefetching and integration with Next.js's routing system, developers can create web applications that stand out in the modern web landscape.