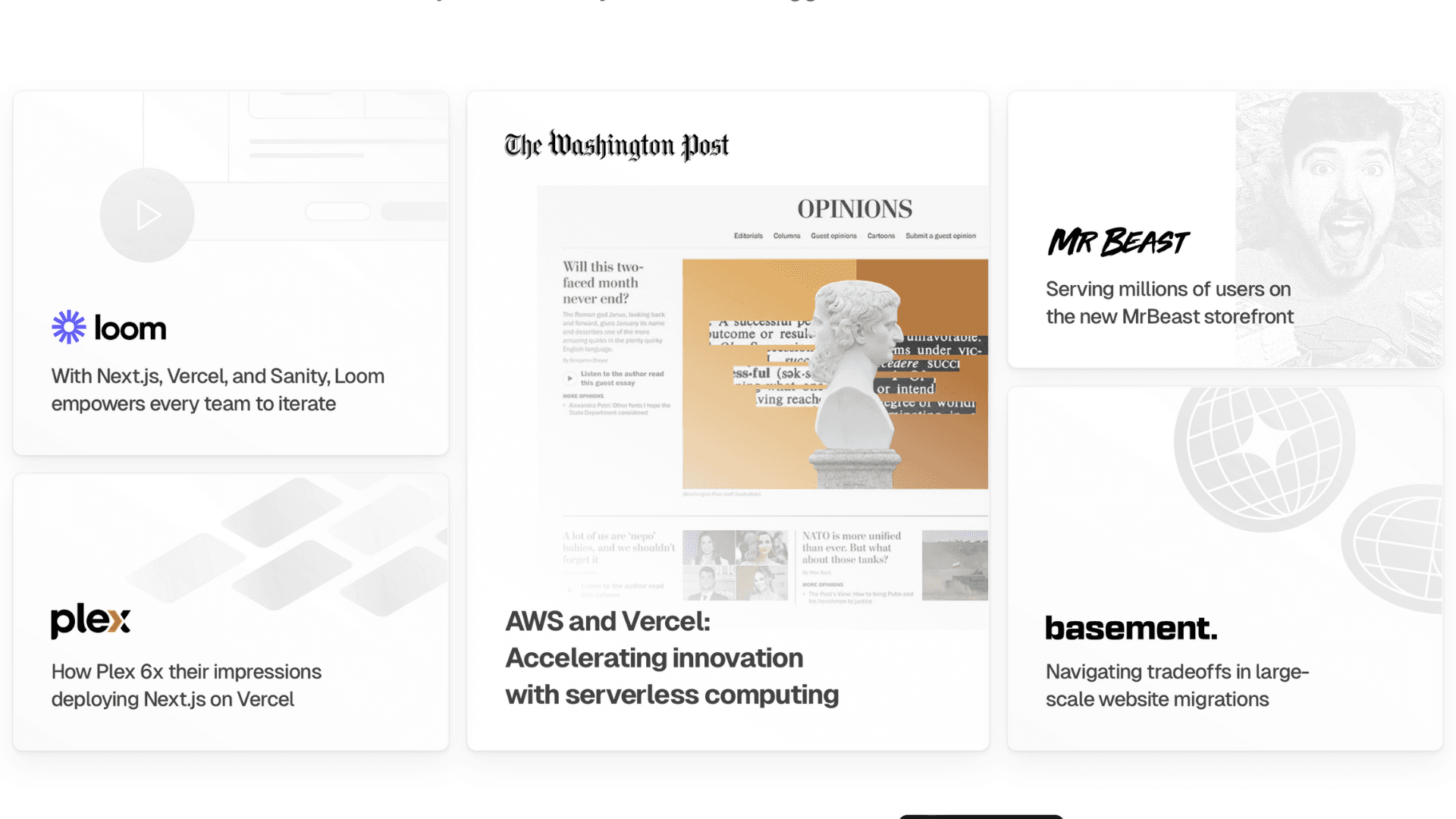
What is the role of the _app.js file in Next.js?
Next.js has emerged as a powerful and popular framework for building React applications, providing a robust set of features for server-side rendering, routing, and more. One essential file that plays a pivotal role in the structure and functionality of a Next.js application is _app.js
. In this article, we will delve into the significance of the _app.js
file and how it influences the overall behavior of a Next.js project.
The _app.js
File:
The _app.js
file is a special file in Next.js that allows developers to customize the way their application is rendered. It acts as a wrapper component for all other pages in the application. This means that any component or layout defined in _app.js
will be present on all pages, providing a centralized place to manage the application's layout, global styles, and other configurations.
Key Responsibilities:
-
Global Layout and Styles: One of the primary responsibilities of the
_app.js
file is to define the global layout for the application. This includes headers, footers, navigation bars, or any other component that should be present on every page. Additionally, global styles, such as CSS imports or styles applied using a CSS-in-JS solution, can be included in this file, ensuring consistent styling across the entire application.// _app.js import "../styles/global.css"; function MyApp({ Component, pageProps }) { // Custom layout or components shared across all pages return <Component {...pageProps} />; } export default MyApp;
-
Customizing the Page Component: The
_app.js
file receives two important props –Component
andpageProps
. TheComponent
prop represents the current page being rendered, andpageProps
contains initial props provided by the Next.js data-fetching methods or external sources. Developers can utilize these props to customize the rendering behavior of pages globally.// _app.js function MyApp({ Component, pageProps }) { // Custom logic or modifications to pageProps return <Component {...pageProps} />; } export default MyApp;
-
Data Fetching and Layout Changes: In addition to customizing the rendering of pages,
_app.js
also enables developers to fetch data or apply layout changes before rendering any page. This is particularly useful for scenarios where global data needs to be loaded or modifications to the layout need to be made on a per-page basis.// _app.js async function MyApp({ Component, pageProps }) { // Custom data fetching or layout modifications const additionalData = await fetchData(); return <Component {...pageProps} additionalData={additionalData} />; } export default MyApp;
-
Lifecycle Methods:
_app.js
supports the use of lifecycle methods, such ascomponentDidMount
orcomponentDidUpdate
, making it a suitable place for handling global events or side effects. This can be especially handy for tasks like initializing analytics, setting up a theme, or managing authentication state.// _app.js class MyApp extends App { componentDidMount() { // Perform global initialization tasks } render() { const { Component, pageProps } = this.props; return <Component {...pageProps} />; } } export default MyApp;
-
Error Handling:
_app.js
is also an excellent place to implement global error handling for your Next.js application. By utilizing error boundaries or implementing custom error-handling logic within this file, you can catch errors that occur anywhere in your application and provide a unified approach to error reporting or logging.// _app.js class MyApp extends App { static getInitialProps({ Component, ctx }) { let pageProps = {}; if (Component.getInitialProps) { try { pageProps = await Component.getInitialProps(ctx); } catch (error) { // Custom error handling logic console.error("Error during getInitialProps:", error); } } return { pageProps }; } render() { const { Component, pageProps } = this.props; return <Component {...pageProps} />; } } export default MyApp;
-
Dynamic Page Routing: If your application requires dynamic routing based on certain conditions,
_app.js
can be instrumental in achieving this. By inspecting thectx
(context) object passed as an argument, you can conditionally redirect users or modify the routing behavior globally.// _app.js class MyApp extends App { static getInitialProps({ Component, ctx }) { // Check user authentication status const isAuthenticated = checkAuthentication(ctx); // Redirect unauthenticated users if (!isAuthenticated && ctx.pathname !== '/login') { if (typeof window === 'undefined') { // Server-side redirect ctx.res.writeHead(302, { Location: '/login' }); ctx.res.end(); } else { // Client-side redirect Router.push('/login'); } } let pageProps = {}; if (Component.getInitialProps) { pageProps = await Component.getInitialProps(ctx); } return { pageProps }; } render() { const { Component, pageProps } = this.props; return <Component {...pageProps} />; } } export default MyApp;
Understanding and leveraging these additional aspects of the _app.js
file empowers developers to create more sophisticated and dynamic Next.js applications. By incorporating error handling, dynamic routing logic, and other global behaviors, developers can ensure a smoother and more controlled user experience across their entire application. This flexibility is one of the key strengths that Next.js provides, making it a versatile framework for building modern web applications.
Conclusion
In summary, the _app.js
file in Next.js plays a central role in defining the global layout, styles, and behavior of a React application. Its flexibility allows developers to implement custom logic, data fetching, and layout modifications that apply universally across all pages. By understanding the role of this file, developers can efficiently structure and manage their Next.js projects, ensuring a consistent and seamless user experience.