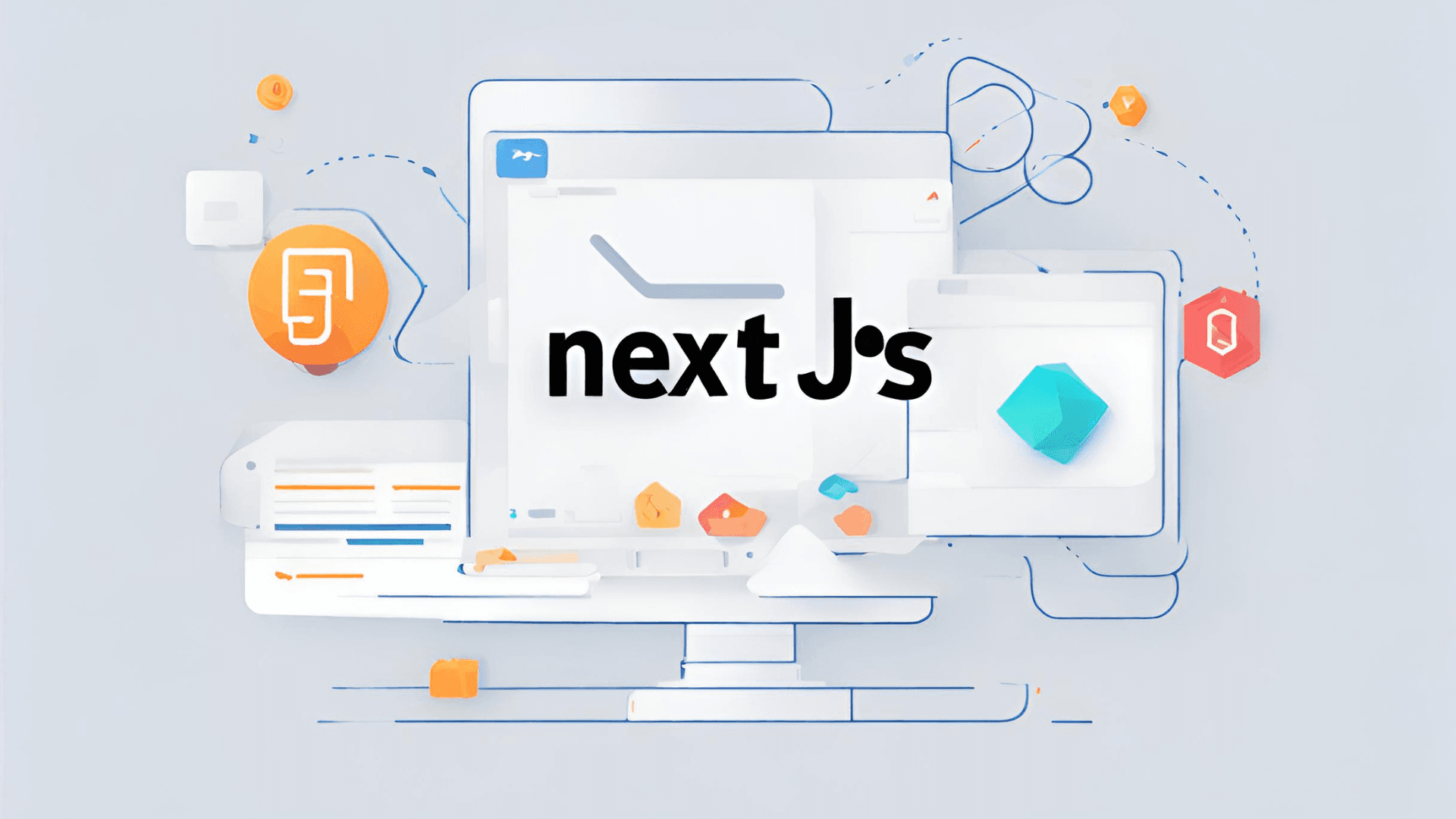
What are the key features of Next.js?
Next.js has emerged as a popular and powerful framework for building React applications, providing developers with a streamlined and efficient way to create modern web experiences. Developed by Vercel, Next.js combines the best features of React with additional tools and optimizations, making it a versatile choice for building dynamic and performant web applications. In this article, we will delve into the key features that make Next.js a preferred framework in the world of web development.
Server-Side Rendering (SSR)
One of the standout features of Next.js is its built-in support for Server-Side Rendering. SSR allows the server to generate HTML on each request, providing a faster initial page load and improving search engine optimization (SEO). With Next.js, achieving SSR is as simple as creating a getServerSideProps
function in your page, giving developers the flexibility to choose which parts of their application benefit from server-side rendering.
// pages/index.js
const HomePage = ({ data }) => {
// Render component with fetched data
};
export async function getServerSideProps() {
// Fetch data on the server
const data = // ...
return {
props: {
data,
},
};
}
export default HomePage;
Static Site Generation (SSG)
Next.js supports Static Site Generation, allowing developers to pre-render pages at build time. This results in faster page loads, reduced server load, and improved user experience. Pages can be generated based on data fetched from APIs, databases, or other sources during the build process.
// pages/about.js
const AboutPage = ({ data }) => {
// Render component with fetched data
};
export async function getStaticProps() {
// Fetch data at build time
const data = // ...
return {
props: {
data,
},
};
}
export default AboutPage;
Automatic Code Splitting
Next.js automatically splits the JavaScript code into smaller chunks, loading only the necessary parts as the user navigates through the application. This results in optimized performance, faster page loads, and a more responsive user experience. Developers can also use dynamic imports to control code splitting explicitly.
// Dynamically import a component
const DynamicComponent = dynamic(
() => import("../components/DynamicComponent"),
);
Routing
Next.js simplifies the process of defining routes within an application. The pages
directory structure automatically maps to the routes, making it intuitive for developers to organize their code. Nested folders in the pages
directory create nested routes.
/pages
/products
/[id].js
In the above structure, a file named [id].js
would handle routes like /products/1
, /products/2
, etc.
API Routes
Next.js provides an easy way to create API routes within the same project. By creating a pages/api
directory, developers can define serverless functions that handle various API endpoints.
// pages/api/user.js
export default function handler(req, res) {
// Handle API request
}
Built-in CSS Support
Next.js comes with built-in support for styling applications, supporting various styling solutions, including CSS, Sass, and CSS-in-JS libraries like styled-components. It allows developers to choose the styling approach that best fits their preferences and project requirements.
Developer-Friendly
Next.js provides a great developer experience with features like fast refresh, which allows for instantaneous feedback during development. The framework also integrates seamlessly with popular tools such as ESLint and TypeScript, making it easy to maintain code quality and use static typing.
Vercel Integration
As the creators of Next.js, Vercel provides seamless integration with their deployment platform. With a single command, developers can deploy their Next.js applications to Vercel, benefiting from features like automatic SSL, serverless functions, and global CDN distribution.
Image Optimization
Next.js simplifies image optimization by automatically handling image loading and compression. The framework supports the next/image
component, which allows developers to optimize images for different devices and screen sizes. It also provides features like lazy loading and automatic generation of responsive image sets, contributing to improved performance and user experience.
// components/ImageComponent.js
import Image from "next/image";
const ImageComponent = () => {
return <Image src="/image.jpg" alt="Description" width={500} height={300} />;
};
Environment Variable Support
Next.js makes it easy to manage environment variables, allowing developers to handle sensitive information or configuration details without hardcoding them into the application. By creating a .env
file and prefixing variables with NEXT_PUBLIC_
, developers can access environment variables in both server-side and client-side code.
// Access environment variable in component
const apiKey = process.env.NEXT_PUBLIC_API_KEY;
TypeScript Support
Next.js has excellent TypeScript integration, enabling developers to use static typing for both server-side and client-side code. TypeScript helps catch potential errors during development, improving code quality and maintainability.
// pages/index.tsx
interface HomePageProps {
data: DataType;
}
const HomePage: React.FC<HomePageProps> = ({ data }) => {
// Render component with fetched data
};
export default HomePage;
Middleware Support
Next.js supports middleware, allowing developers to execute code before handling a request. This is useful for tasks such as authentication, logging, or modifying the request and response objects. Middleware functions can be added to the pages/api
directory.
// pages/api/middleware.js
export default function middleware(req, res, next) {
// Execute middleware logic
next();
}
// pages/api/user.js
import middleware from './middleware';
export default function handler(req, res) {
// Handle API request
}
// Apply middleware to the user API route
export const config = {
api: {
bodyParser: false,
},
};
Internationalization (i18n)
Next.js provides built-in support for internationalization, making it easy for developers to create applications that support multiple languages. By configuring the next.config.js
file and using the next-translate
library, developers can manage translations and provide a localized experience for users.
// pages/index.js
import useTranslation from "next-translate/useTranslation";
const HomePage = () => {
const { t } = useTranslation("common");
return (
<div>
<p>{t("welcomeMessage")}</p>
</div>
);
};
Next.js continues to evolve and enhance the development experience, offering a robust set of features that cater to various project requirements. Its flexibility, performance optimizations, and developer-friendly environment make it a compelling choice for building modern web applications. As web development standards and practices evolve, Next.js remains at the forefront, providing developers with the tools they need to create powerful and efficient web experiences. Whether you are a seasoned developer or just starting, Next.js is certainly a framework worth exploring for your next project.
Next.js has become a go-to framework for many developers building React applications due to its powerful features, ease of use, and performance optimizations. Whether it's server-side rendering, static site generation, or automatic code splitting, Next.js provides a comprehensive solution for building modern, high-performance web applications. As web development continues to evolve, Next.js remains at the forefront, empowering developers to create robust and efficient user experiences.
Frequently Asked Questions about Next.js
1. What is Next.js?
Next.js is a React framework that enables features like server-side rendering, static site generation, and hybrid applications. It is designed for building fast, user-friendly web applications and websites with minimal configuration.
2. What is the difference between Server-Side Rendering (SSR) and Static Site Generation (SSG) in Next.js?
- SSR: Pages are generated on the server at request time, meaning the HTML is dynamically generated for each request, improving SEO and initial page load times.
- SSG: Pages are pre-rendered at build time, meaning the HTML is generated once and served for all subsequent requests, making it ideal for static content that doesn't change frequently.
3. What is Incremental Static Regeneration (ISR)?
ISR allows you to update or regenerate specific static pages after they are built, without needing to rebuild the entire site. This feature is great for content that changes periodically, as it combines the benefits of static sites with dynamic updates.
4. What are API Routes in Next.js?
API Routes in Next.js allow you to create serverless API endpoints within your application. This means you can handle backend logic, such as form submissions, data fetching, or integrations, directly in the app without needing a separate backend.
5. How does Next.js handle routing?
Next.js uses a file-based routing system. Every file inside the pages
directory automatically becomes a route in the application. This makes routing simple and intuitive compared to traditional React apps, where routing is handled manually.
6. How does Next.js optimize images?
Next.js includes built-in image optimization. The <Image>
component automatically serves the most appropriate image size and format (like WebP) based on the device, optimizing load times and improving performance.
7. What is Fast Refresh in Next.js?
Fast Refresh allows you to see updates to your code in real-time without reloading the entire page or losing the current state of your components. It enhances the development experience by making the feedback loop quicker.
8. Does Next.js support TypeScript?
Yes, Next.js has first-class TypeScript support. You can easily create a TypeScript project by simply adding .ts
or .tsx
files, and Next.js will automatically configure the necessary TypeScript settings for your project.
9. What is Automatic Code Splitting?
Automatic Code Splitting in Next.js ensures that only the necessary JavaScript is loaded for the page being viewed. This reduces load times and improves overall performance by splitting the code into smaller, more manageable chunks.
10. Can I customize the configuration in Next.js?
Yes, Next.js allows for customization through a next.config.js
file. You can configure settings like Webpack, Babel, environment variables, and more to tailor the project to your specific needs.
11. How does Next.js compare to Create React App (CRA)?
Next.js offers more advanced features like SSR, SSG, and ISR, while CRA is more suitable for single-page applications with client-side rendering. Next.js is a better choice for larger applications or websites that require strong SEO and performance optimizations.
Here are some useful references to deepen your understanding of Next.js and its features:
-
Official Next.js Documentation
The best place to start for an in-depth understanding of Next.js. It covers everything from basic setup to advanced topics like SSR, SSG, and API routes.
Next.js Docs -
Next.js GitHub Repository
The official source code of Next.js. Browsing through the repository can give you insights into its development, new releases, and community contributions.
Next.js GitHub -
Vercel Blog
Vercel (the creators of Next.js) frequently posts updates, tutorials, and best practices for using Next.js in production.
Vercel Blog -
Next.js Learn
An interactive tutorial by Vercel that walks you through building a Next.js project from scratch, covering the fundamentals and key features.
Learn Next.js -
CSS Tricks: Understanding Next.js
A beginner-friendly introduction to Next.js and its ecosystem, covering essential concepts in a straightforward manner.
Understanding Next.js on CSS Tricks -
YouTube: Next.js Crash Course by Traversy Media
A detailed crash course that covers the basics of Next.js in a practical, hands-on way. Great for visual learners who want to see real-world coding examples.
Next.js Crash Course -
Dev.to: Advanced Next.js Techniques
Articles and blog posts from the developer community on using advanced features of Next.js, including dynamic routing, API routes, and more.
Advanced Next.js on Dev.to -
Next.js Examples
A collection of Next.js example projects that demonstrate the use of different features and integrations. You can explore and clone these examples to get started quickly.
Next.js Examples
These resources provide a comprehensive foundation for both beginners and advanced developers to master Next.js.