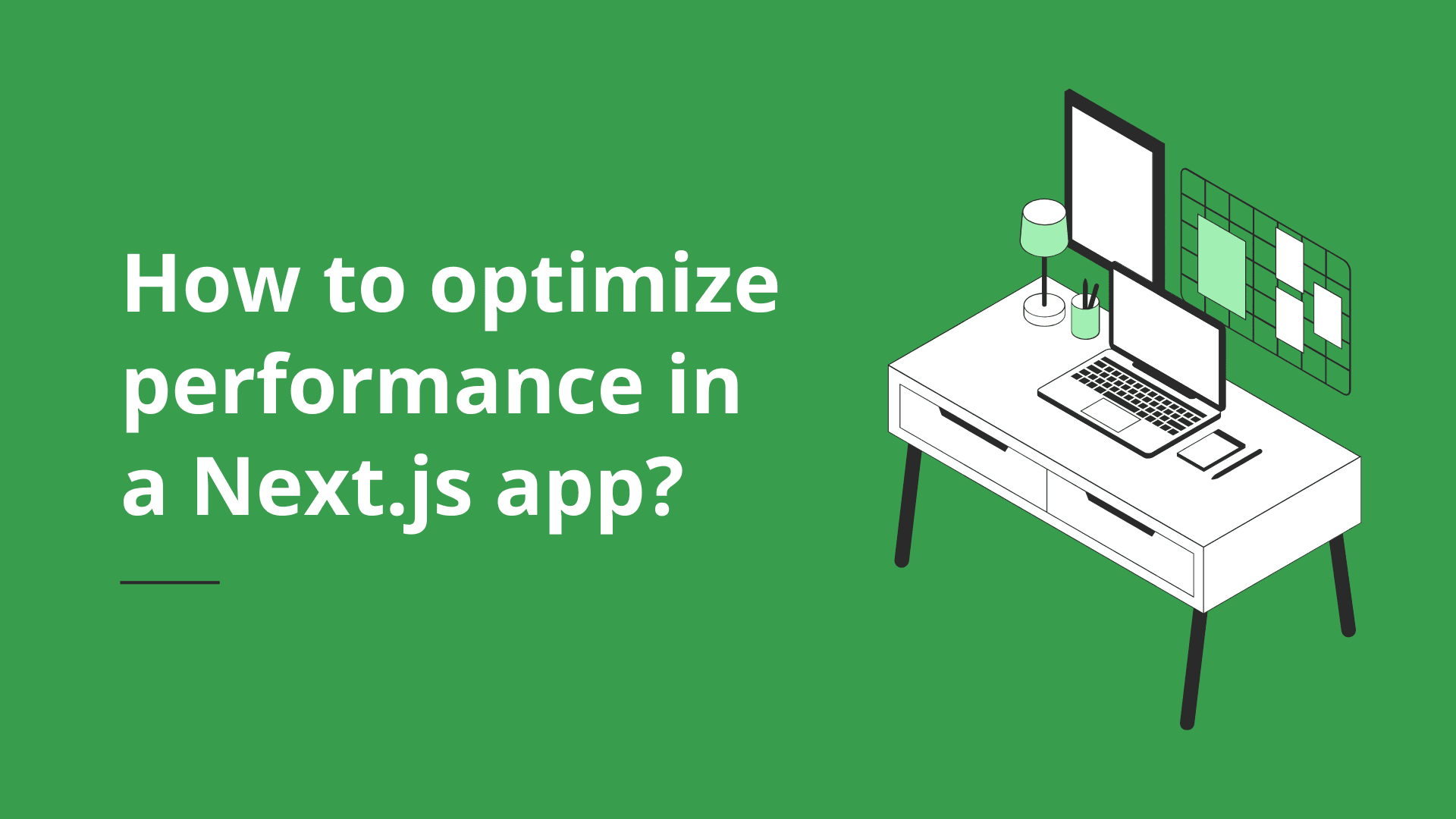
How to optimize performance in a Next.js app?
Optimizing the performance of a Next.js app is crucial for enhancing user experience, improving search engine rankings, and maintaining a competitive edge. Next.js, a popular React framework, offers several built-in features and best practices for optimizing app performance. Here's a detailed guide on how to do it:
➾ Leverage Static Generation and Server-Side Rendering
Next.js allows you to pre-render pages using Static Generation and Server-Side Rendering, which can significantly improve the load time of your pages.
- Static Generation (SG): Use SG for pages that do not require real-time data. It generates HTML at build time, making the page loading extremely fast.
- Server-Side Rendering (SSR): For pages that need real-time data, SSR is a better choice. It generates HTML on each request, ensuring the data is up-to-date.
➾ Optimize Images
Images often account for the majority of a webpage's size. Next.js provides an <Image />
component that automatically optimizes images.
- Use the
<Image />
component fromnext/image
to serve images in optimized formats, resize images on-the-fly, and only load images when they enter the viewport (lazy loading). - Ensure you specify the
width
andheight
attributes to maintain aspect ratio and avoid layout shifts.
➾ Code Splitting and Dynamic Imports
Next.js automatically splits your code at the component level, but you can further optimize performance by dynamically importing modules that are not immediately necessary.
- Use dynamic imports (
import()
) for components and libraries that are only required under certain conditions or on user interaction. - This reduces the initial bundle size, leading to faster page loads.
➾ Eliminate Unused Code
- Tree Shaking: Next.js automatically supports tree shaking, eliminating unused code from your final bundle.
- Custom Babel Plugins: Use Babel plugins to remove or replace code that isn't needed in production.
➾ Use the Built-in ESLint Configuration
Next.js comes with an integrated ESLint setup that can help identify performance issues, such as missing key
props in lists or images without alt
text.
- Regularly run ESLint to catch and fix common issues that could affect performance.
➾ Implement Incremental Static Regeneration (ISR)
ISR allows you to update static content after the site has been built without needing to rebuild the entire site.
- Use ISR for pages that need to be updated frequently but don't require real-time data. This provides the benefits of static generation with near real-time updates.
➾ Optimize Third-Party Scripts and CSS
- Load third-party scripts asynchronously or defer their loading to ensure they don't block the main thread.
- Minimize and optimize CSS, and consider using CSS modules or styled-components to scope CSS to components, reducing unused styles.
➾ Prioritize Above-the-Fold Content
- Use the
priority
prop on the<Image />
component for above-the-fold images to hint their importance. - Consider custom loading strategies for critical CSS and JavaScript that impact above-the-fold content.
➾ Use the Web Vitals API
Next.js integrates with the Web Vitals API, allowing you to measure and analyze real user performance metrics.
- Monitor Core Web Vitals (Largest Contentful Paint, First Input Delay, and Cumulative Layout Shift) to identify and address performance issues.
➾ Deploy on a Global CDN
- Use a provider that deploys your Next.js app on a global CDN. This reduces the distance between your users and the server, decreasing load times.
➾ Enable Compression
Compression reduces the size of your files before they're sent over the network, which can significantly decrease load times for users.
- Ensure your server is configured to use compression methods like Gzip or Brotli for serving JavaScript, CSS, and HTML files. Next.js supports automatic static optimization, but for dynamic responses, you may need to configure compression at the server level or use a hosting solution that does this automatically.
➾ Optimize Fonts
Web fonts can be a significant contributor to page load times. Optimize the use of fonts through the following strategies:
- Use
font-display: swap;
in your CSS to ensure text is visible during font loading. - Preload your most important fonts to tell the browser to download them as soon as possible.
- Subset fonts to include only the characters you need.
- Use variable fonts where possible to reduce the number of font files needed.
➾ Use the next/script
Component for Third-Party Scripts
Next.js offers a <Script />
component that optimizes loading third-party scripts. It allows you to control when and how scripts are loaded, minimizing their impact on performance.
- Utilize the
strategy
attribute to define how scripts should be loaded (beforeInteractive
,afterInteractive
,lazyOnload
). - This ensures that non-essential scripts don't interfere with the critical loading path.
➾ Cache Assets
Caching can significantly reduce load times for repeat visitors.
- Leverage HTTP Caching: Configure your server to provide appropriate
Cache-Control
headers for your assets. This tells browsers how long to cache assets. - Service Workers: Implement service workers to cache assets on the client-side, providing offline support and faster load times on subsequent visits.
➾ Analyze and Monitor Bundle Size
Keeping an eye on your bundle size is crucial for maintaining fast load times.
- Use Next.js's built-in analytics to monitor the size of your JavaScript bundles.
- Employ tools like Webpack Bundle Analyzer to understand what's contributing to your bundle size and identify opportunities for optimization.
➾ Database and API Response Optimization
If your application relies on data from APIs or databases, optimizing these responses can have a substantial impact on performance.
- Reduce API Payloads: Only fetch the data that is needed for rendering the page.
- Use Caching: Implement caching strategies for API responses to reduce the number of requests to your server or external APIs.
- Optimize Database Queries: Ensure that your database queries are efficient and fast. Indexing, query optimization, and database caching can lead to significant performance improvements.
➾ Static Assets Versioning
Versioning static assets can help with cache management. By appending a version number or hash to file names (e.g., style.v2.css
), you can invalidate the browser cache when files are updated, ensuring users always receive the most recent version.
➾ Use Environment Variables for Configuration
Next.js supports environment variables, which can be useful for toggling between development and production configurations without code changes. This can include enabling/disabling analytics, feature flags, or other optimizations that should only run in production.
➾ Leverage Incremental Adoption
Next.js supports incremental adoption, allowing you to gradually introduce Next.js into an existing application or to migrate from another framework. This approach lets you optimize performance piece by piece, without the need for a complete rewrite.
- Start with high-traffic pages: Focus on optimizing and migrating high-traffic or performance-critical pages to Next.js first. This can provide immediate benefits in terms of performance and user experience.
➾ Optimize Custom Server Usage
If you're using a custom server with Next.js, be cautious, as it can impact performance. The custom server functionality allows for additional customization at the expense of some optimizations that Next.js provides out of the box.
- Keep it lean: Only add necessary customizations to your server setup to avoid negating Next.js's built-in performance benefits.
- Consider serverless: For many use cases, deploying on a serverless platform can automatically scale and optimize your application's performance without the need for a custom server.
➾ Adopt Progressive Web App (PWA) Techniques
Turning your Next.js application into a PWA can improve its performance, especially on mobile devices. PWAs can utilize service workers, cache assets effectively, and even function offline.
- Implement service workers: Use service workers to cache the shell of your application and other assets, which can dramatically improve load times and provide offline capabilities.
- Add a web app manifest: This allows users to add your site to their home screen for quick access, providing a more app-like experience.
➾ Fine-tune React Components
Since Next.js is built on top of React, optimizing your React components can also boost your application's performance.
- Avoid unnecessary re-renders: Use React's
React.memo
,useMemo
, anduseCallback
to prevent unnecessary re-renders of components. - Profile component performance: Use React Developer Tools to profile your application and identify slow components.
➾ Implement Security Best Practices
While not directly related to performance, ensuring your Next.js application is secure can prevent performance issues down the line, such as those caused by malicious attacks or data breaches.
- Sanitize user input: Always sanitize user input to prevent XSS attacks, which can inject unwanted scripts into your pages.
- Use HTTPS: Serve your Next.js application over HTTPS to secure data in transit.
- Keep dependencies updated: Regularly update your Next.js and other dependencies to patch known vulnerabilities.
➾ Engage the Community and Resources
The Next.js community is an excellent resource for performance tips, plugins, and best practices. Engaging with the community can provide insights and strategies for optimizing your application.
- Follow the Next.js GitHub repository and discussions for updates and performance-related conversations.
- Explore plugins and integrations that can enhance your app's performance. The Next.js ecosystem is rich with tools designed for specific performance optimizations.
➾ Continuous Performance Testing
Finally, establishing a routine for continuous performance testing ensures that your optimizations are effective and that new updates do not degrade performance over time.
- Integrate performance testing into your CI/CD pipeline: Tools like Lighthouse, WebPageTest, or custom scripts can automatically test your application's performance on each commit or release.
- Monitor real-user metrics (RUM): Tools like Google Analytics, Vercel Analytics, or other third-party services can provide insights into how real users experience your application across different devices and networks.
➾ Minimize External Requests
Each external request your application makes can add to the load time. This includes requests for scripts, stylesheets, fonts, and APIs.
- Bundle external resources locally: Where possible, serve third-party scripts or libraries from your own server.
- Combine CSS and JavaScript files: Although Next.js does an excellent job of bundling, ensure that custom scripts or stylesheets are combined and minified to reduce requests.
➾ Optimize Third-Party Integrations
While third-party integrations (like analytics, chatbots, or customer support widgets) can add valuable functionality to your Next.js app, they can also impact performance.
- Evaluate the impact of each integration: Use performance tools to measure how each third-party script affects load times and evaluate whether its utility outweighs the performance cost.
- Lazy load non-essential integrations: Delay the loading of non-critical third-party scripts until after the main content has loaded or until they are needed.
➾ Adopt HTTP/2 and HTTP/3
Modern HTTP protocols like HTTP/2 and HTTP/3 offer performance improvements over HTTP/1.1, including multiplexing and header compression.
- Ensure your server and CDN support HTTP/2 or HTTP/3: These protocols can reduce latency and improve load times by optimizing the transfer of data between clients and servers.
➾ Utilize Client-Side Caching Strategies
In addition to server-side caching, implementing client-side caching can further enhance your application's performance.
- Service Workers: Beyond PWAs, service workers can be used for fine-grained caching strategies, intercepting network requests, and serving cached responses.
- IndexedDB: For complex data or large datasets, consider using IndexedDB to store data on the client side, reducing the need for repeated API calls.
➾ Optimize API Calls
Making efficient API calls is crucial for application performance, especially for applications that rely heavily on dynamic data.
- Batch requests: Minimize the number of API calls by batching requests together, if possible.
- Debounce and throttle user inputs: For features like search or auto-complete, implement debouncing or throttling to limit API calls based on user input.
➾ Monitor and Optimize the Critical Rendering Path
The Critical Rendering Path is the sequence of steps the browser goes through to convert HTML, CSS, and JavaScript into pixels on the screen. Optimizing this path is crucial for reducing First Contentful Paint (FCP) and Time to Interactive (TTI).
- Minimize critical CSS: Identify and inline the CSS necessary for rendering the above-the-fold content, and defer non-critical CSS.
- Asynchronous loading: Load JavaScript files asynchronously to prevent them from blocking the DOM rendering.
➾ Adaptive Loading
Implement adaptive loading in your Next.js app to tailor the experience based on the user's device capabilities and network conditions.
- Responsive images: Utilize the
sizes
andsrcset
attributes in the<Image />
component to serve images best suited to the user's device. - Network-aware loading: Adjust the quality and quantity of content based on the user's network speed, potentially reducing the fidelity of images or deferring non-critical resources on slower connections.
➾ A/B Testing for Performance
When implementing performance optimizations, A/B testing can help you understand the impact of changes on real-world user experiences.
- Split testing: Use split testing to compare the performance of two versions of a page or feature under real conditions.
- Iterative optimization: Use the insights gained from A/B testing to make informed decisions about further optimizations and refinements.
➾ Accessibility Considerations
Performance optimizations should go hand in hand with accessibility improvements. A fast site that's inaccessible fails to serve a significant portion of potential users.
- Semantic HTML: Use semantic HTML elements for better screen reader compatibility, which can also improve SEO.
- Accessible loading indicators: Provide accessible feedback for any lazy-loaded content or asynchronous operations, ensuring that all users are aware of ongoing processes.
➾ Optimize Image Loading with Placeholders
Image loading can significantly impact performance, especially on image-heavy websites. Implementing placeholders can improve the perceived performance.
- Skeleton screens: Use skeleton screens as placeholders to indicate where images or content will load. This improves the perceived loading time as users see a page layout immediately, even before the content fully loads.
- Blur-up technique: Initially load a tiny, blurred version of the image that's quickly replaced by the full-resolution image once it's loaded. This technique can be easily implemented with Next.js's
Image
component using theplaceholder="blur"
attribute.
➾ Prioritize User Interactions
Ensuring that the website remains responsive to user inputs, even as resources are being loaded, is crucial for a good user experience.
- Split interactive code: Use dynamic imports to split off the code handling user interactions from the main bundle. This ensures that interactive elements become responsive more quickly.
- Use web workers for heavy computations: Offload heavy computations to web workers to keep the main thread free for user interactions.
➾ Implement Predictive Prefetching
Predictive prefetching involves loading resources you anticipate the user will need next, based on their current interactions.
- Link prefetching: Next.js supports prefetching pages out of the box with the
<Link>
component'sprefetch
prop. Consider strategically prefetching pages based on user behavior patterns. - Data prefetching: For dynamic content, prefetch data for the next probable user actions. For instance, if a user is reading an article, prefetch data for the next article in the series.
➾ Optimize Third-Party CSS
Third-party libraries and frameworks can add a significant amount of CSS to your project, much of which might not be used.
- Tree-shake CSS: Use tools and techniques to remove unused CSS from your bundles. Tools like PurgeCSS can help automate this process for third-party libraries.
- Critical CSS: Extract and inline critical CSS for above-the-fold content, deferring the rest.
➾ Environment-Specific Optimizations
Different environments (development, staging, production) have different optimization needs. Tailor your Next.js configuration and optimizations for each environment.
- Dynamic configurations: Utilize Next.js's support for environment variables to toggle features, optimizations, and logging based on the current environment.
- Enable profiling in development: Use React's Profiler in development to catch performance issues early, without affecting production performance.
➾ Monitor Web Vitals
Core Web Vitals are a set of specific factors that Google considers important in a webpage's overall user experience. Next.js has built-in support for measuring these vitals.
- Leverage Next.js Analytics: Use Vercel's Next.js Analytics to get real-time feedback on Core Web Vitals across your application.
- Continuous monitoring: Implement tools to continuously monitor these vitals, even post-launch, to understand how real-world usage impacts performance.
➾ SEO Considerations
Performance optimizations often align with SEO best practices, as search engines favor fast, efficient websites.
- Structured data: Use schema.org markup to help search engines understand your content better, potentially leading to better indexing and performance.
- Optimize for mobile: Ensure your Next.js application is fully responsive and optimized for mobile devices, as mobile-friendliness is a significant factor in search engine rankings.
➾ Content Delivery Network (CDN)
A CDN can dramatically improve load times for your static assets and media by serving content from a location nearest to the user.
- Choose a CDN that integrates well with Next.js: Vercel, the platform behind Next.js, offers a CDN out of the box, but there are many options available that can be configured to work with Next.js applications.
➾ Automate Performance Testing
Automate your performance testing to catch regressions and improvements over time.
- Integrate with CI/CD: Tools like Lighthouse CI can be integrated into your CI/CD pipeline to automatically run performance tests on each commit or pull request.
- Regular audits: Schedule regular performance audits to ensure that your optimizations remain effective as your application evolves.
➾ Optimize Fonts Loading
Fonts can significantly affect your site's perceived performance. Optimizing how fonts are loaded and displayed can reduce layout shifts and improve content readability faster.
- Use
font-display
: Utilize thefont-display
CSS property to control how and when fonts are displayed. For example,font-display: swap;
ensures text is immediately visible with a fallback font while the custom font loads. - Preload key fonts: Preload your most important fonts using
<link rel="preload" href="/path/to/font.woff2" as="font" type="font/woff2" crossorigin>
in your document's<head>
. This tells the browser to prioritize downloading this font early in the page load process. - Self-host fonts: Where possible, self-host fonts to avoid additional DNS lookups, connection overheads, and potential privacy issues associated with third-party font hosting services.
➾ Use the Built-in Next.js Internationalization (i18n) Routing
If your application targets users in multiple countries or languages, implementing internationalization correctly is crucial for both performance and user experience.
- Leverage Next.js i18n: Next.js offers built-in support for internationalized routing. This feature automatically handles the loading of the appropriate language files based on the route, which can significantly simplify and speed up the implementation of internationalization.
- Lazy load translations: Load only the necessary translations for the user's current language, rather than loading all translation files upfront. This can reduce the initial load time and save bandwidth.
➾ Utilize Environment Variables for Configuration
Managing configurations across different environments (development, staging, production) can be challenging. Environment variables allow you to customize the Next.js app's behavior without changing the code.
- Next.js environment variables: Use
.env.local
,.env.development
,.env.production
, etc., to configure environment-specific variables. This approach keeps your configuration secure and separate from your application code. - Runtime configuration: For variables that need to be exposed to the browser, use Next.js's runtime configuration feature with caution to avoid exposing sensitive information.
➾ Continuous Integration (CI) and Continuous Deployment (CD) Optimization
CI/CD pipelines can be optimized to improve build times and ensure that performance optimizations are correctly applied before deployment.
- Cache dependencies: Configure your CI/CD pipeline to cache
node_modules
or other dependencies between builds. This can significantly reduce installation time. - Parallelize tasks: Where possible, run tests, linting, and builds in parallel to speed up the CI process.
- Selective builds: Use monorepo strategies or custom scripts to build and deploy only the parts of your application that have changed, rather than rebuilding the entire application.
➾ Implement Server-Side Caching Strategies
Server-side caching can dramatically improve response times for dynamic content by avoiding unnecessary database queries or computation.
- HTML caching: Cache fully rendered HTML pages at the server level to serve them quickly on subsequent requests.
- Data caching: Implement caching for frequently accessed data, such as API responses or database queries. Use a caching layer like Redis or memcached for efficient data retrieval.
- Cache invalidation: Implement a robust cache invalidation strategy to ensure that users always receive up-to-date content.
➾ Security and Performance
A secure application is inherently more performant, as it's less likely to be slowed down by malicious attacks or data breaches.
- Implement Content Security Policy (CSP): CSP helps prevent XSS attacks by controlling the resources allowed to load on your pages, potentially reducing the risk of including malicious or unnecessary external scripts.
- Rate limiting: Protect your APIs and servers from abuse and denial-of-service attacks by implementing rate limiting. This can prevent your system from being overwhelmed by too many requests, ensuring availability and performance for legitimate users.
➾ Community and Contribution
Being part of the Next.js and wider web development community can provide access to new ideas, optimizations, and best practices. Contributing back can also help improve the ecosystem.
- Stay engaged with the community: Participate in forums, attend meetups, and contribute to discussions. The insights gained can inform your optimization strategies.
- Contribute to open source: If you develop solutions, plugins, or optimizations that could benefit others, consider contributing to the Next.js community or related projects. Open source contributions can help improve the tools and frameworks everyone relies on.
Conclusion
Optimizing a Next.js application is a continuous and evolving process that encompasses various aspects of web development, including coding practices, build and deployment strategies, and user experience considerations. By adopting a holistic approach to performance optimization, regularly testing and monitoring your application, and staying engaged with the development community, you can ensure that your Next.js app remains fast, efficient, and competitive in the ever-changing landscape of web technology.