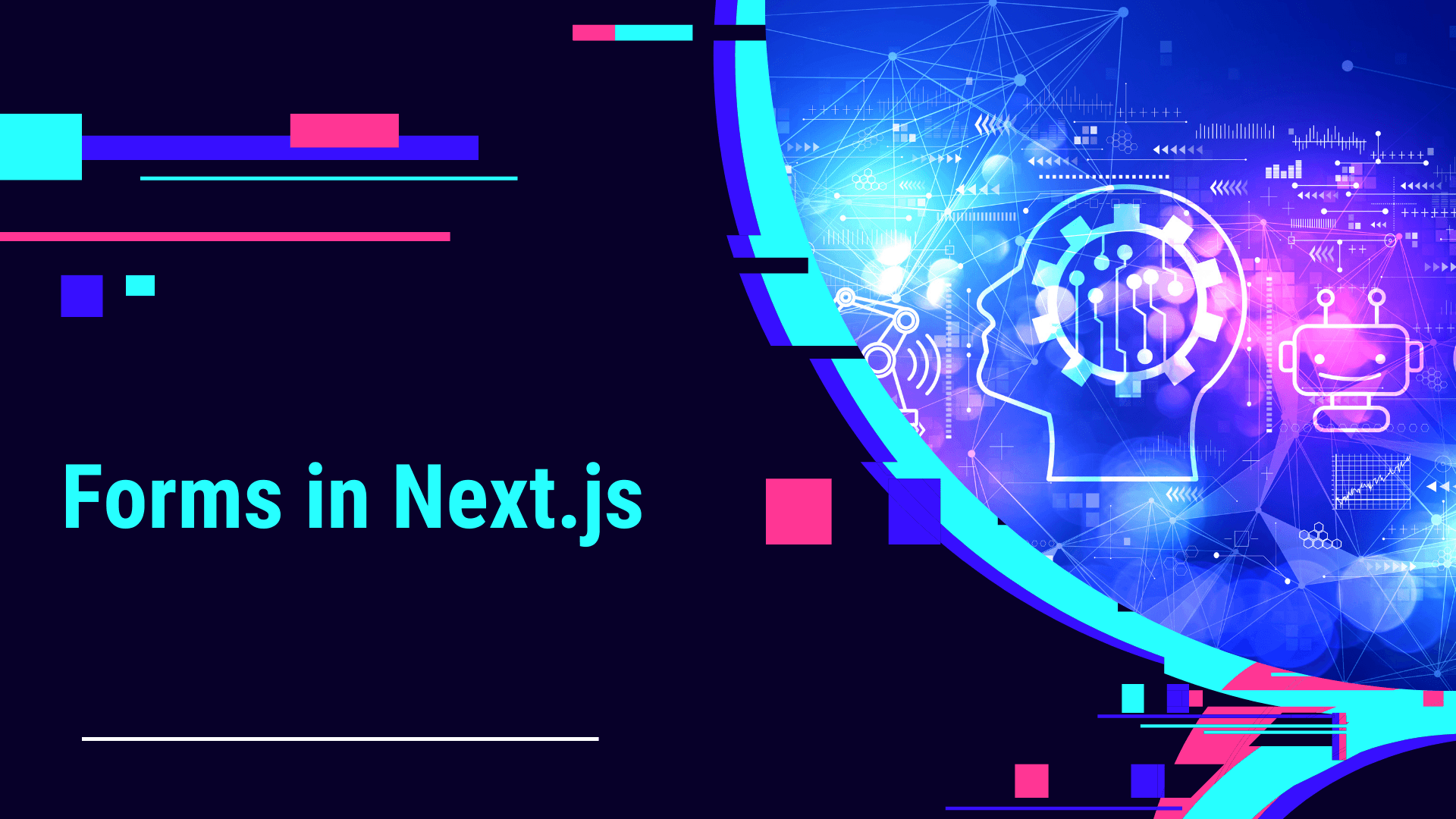
How to handle forms in a Next.js application?
Handling forms in a Next.js application can be a critical aspect of building interactive and user-friendly web applications. Next.js, a popular React framework, offers several ways to manage form data, submit forms, and handle server-side processing. This article will guide you through the best practices for handling forms in Next.js, covering everything from the basics of form creation to handling submissions and integrating with back-end services.
Creating a Form in Next.js
The first step is creating the form itself. In Next.js, you can use standard HTML <form>
elements combined with React state to manage form inputs. Here's a simple example:
import { useState } from "react";
export default function ContactForm() {
const [email, setEmail] = useState("");
const handleSubmit = (event) => {
event.preventDefault();
// Handle the form submission here
console.log(email);
};
return (
<form onSubmit={handleSubmit}>
<label htmlFor="email">Email:</label>
<input
type="email"
id="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
<button type="submit">Submit</button>
</form>
);
}
Form Validation
Client-side validation is crucial for improving user experience by providing immediate feedback. You can implement validation using HTML5 form attributes like required
, minLength
, maxLength
, and pattern
or by manually checking the values in your submission handler before proceeding with the form submission.
For more complex validation scenarios, consider using a library like Yup or Joi alongside form libraries like Formik or React Hook Form, which significantly simplify the process.
Handling Form Submission
Handling form submissions involves capturing the form data and possibly sending it to a server. In the example above, the handleSubmit
function is responsible for this. For server communication, you might use the Fetch API or Axios to send the data via HTTP.
const handleSubmit = async (event) => {
event.preventDefault();
try {
const response = await fetch("/api/contact", {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({ email }),
});
// Handle response
console.log(await response.json());
} catch (error) {
console.error(error);
}
};
Server-side Handling in Next.js
Next.js allows you to handle form submissions on the server side using API routes. Create a file under pages/api
to handle the POST request. For example, pages/api/contact.js
could look like this:
export default function handler(req, res) {
if (req.method === "POST") {
// Process POST request
const { email } = req.body;
// Perform server-side operations, like sending an email or saving to a database
res.status(200).json({ message: "Success", email });
} else {
// Handle any other HTTP methods
res.setHeader("Allow", ["POST"]);
res.status(405).end(`Method ${req.method} Not Allowed`);
}
}
Managing State and Server-side Validation
For complex forms, managing state and validations can become cumbersome. Libraries like Formik or React Hook Form can help manage form state, handle submissions, and validate inputs with ease. They also integrate well with validation schemas from Yup or Joi, offering a comprehensive solution for form handling in React and Next.js applications.
Security Considerations
Always validate and sanitize form inputs on the server side to prevent SQL injection, cross-site scripting (XSS), and other security vulnerabilities. Additionally, consider implementing CSRF (Cross-Site Request Forgery) protection for your forms.
Enhancing User Experience with Form Handling
Creating a great user experience involves more than just efficiently collecting and processing data. Feedback mechanisms, loading states, and error handling play critical roles in informing users about what's happening and any steps they need to take. Let's delve deeper into these aspects:
Providing Real-time Feedback
Real-time feedback helps users correct mistakes immediately, without waiting for a server response. Implementing inline validation and providing helpful error messages can significantly enhance the user experience. For example, if an input field requires an email, you can validate the user's input as they type and show a message if the input doesn't match the expected pattern.
Managing Loading States and Success Messages
When a form is submitted, it's essential to provide feedback to the user that something is happening, especially if the network request to your server might take a few seconds. Changing the submit button to a loading state or displaying a spinner are common ways to indicate that the submission is in progress.
After a successful submission, providing a clear success message or redirecting the user to a confirmation page can help close the loop, letting them know that their action was successful.
const [loading, setLoading] = useState(false);
const [message, setMessage] = useState("");
// Inside your submit handler
setLoading(true);
fetch("/api/contact", {
method: "POST",
// Additional fetch options...
})
.then((response) => response.json())
.then((data) => {
setMessage(data.message); // "Success"
setLoading(false);
})
.catch((error) => {
console.error(error);
setLoading(false);
});
// In your return statement
<button type="submit" disabled={loading}>
{loading ? "Submitting..." : "Submit"}
</button>;
{
message && <p>{message}</p>;
}
Error Handling
Error handling is another critical aspect of form management. If the server responds with an error (e.g., due to validation failures or server issues), you should display these errors to the user so they can take corrective action.
For complex forms, consider mapping server-side errors back to specific form fields and displaying contextual messages. This approach requires a consistent error response format from your server-side API.
Optimizing for Accessibility
Accessibility (a11y) is crucial for making your forms usable by as many people as possible, including those using screen readers or other assistive technologies. Ensure your forms are accessible by:
- Using semantic HTML elements and attributes (e.g.,
<label for="...">
,aria-
attributes). - Providing clear and descriptive labels and error messages.
- Ensuring keyboard navigability.
State Management for Complex Forms
For applications with complex forms or multiple forms, consider using a global state management solution like React Context or Redux to manage form states. This approach can simplify the passing of form data and errors between components, especially in large applications.
Embracing Best Practices
Handling forms in Next.js, or any web framework, requires attention to detail and a focus on user experience. By leveraging Next.js features, such as API routes for server-side processing, and incorporating best practices in form design, validation, and submission handling, developers can create efficient, secure, and user-friendly forms. Remember, the goal is not just to collect data but to ensure a seamless and accessible experience for all users.
Optimizing Performance
As your Next.js application grows, especially with complex forms on multiple pages, performance optimization becomes crucial. Efficient form handling can contribute significantly to faster page loads and smoother user interactions. Here are strategies to keep your forms performing well:
- Lazy Loading Components: Use dynamic imports with
React.lazy
for splitting your form components and only loading them when needed. Next.js also supports dynamic imports out of the box, which can be used to optimize the loading of components. - Memoization: Utilize
React.memo
for form components that do not change frequently. This prevents unnecessary re-renders, reducing the computational load. - State Management Optimization: Be mindful of where and how you manage state. Local state management within forms is often more performance-efficient than global state management for data that doesn't need to be shared across the entire app.
Accessibility (a11y) Enhancements
Building on the basics of form accessibility, further enhancements ensure that your forms are not only compliant with standards like WCAG (Web Content Accessibility Guidelines) but also provide a superior user experience for people with disabilities:
- Focus Management: Ensure that navigating through the form is intuitive, especially for users relying on keyboards or screen readers. Managing focus on input fields, error messages, and after form submission can greatly enhance usability.
- Semantic HTML: Use the correct HTML elements for your form and its components. For example, fieldsets, legends, and labels improve the structure and interpretability of your forms.
- ARIA Attributes: When standard HTML elements fall short, use ARIA (Accessible Rich Internet Applications) attributes to provide additional context to assistive technologies.
Progressive Enhancement
Consider progressive enhancement strategies for your forms. This means building your core form functionality using simple HTML and then enhancing it with CSS and JavaScript. This approach ensures that your form remains functional even if JavaScript fails to load or is disabled by the user, enhancing reliability and accessibility.
User Feedback and Iteration
Collecting user feedback on your forms can unveil insights into how real users interact with them, what issues they face, and what improvements can make their experience better. Tools like Hotjar or FullStory can help you understand user behavior, while direct feedback can be collected through surveys or feedback forms.
Iterate on your forms based on this feedback and analytics data to continuously improve the user experience. A/B testing different form designs and flows can also help identify what works best for your audience.
Keeping Up with Next.js and Web Standards
Next.js and web technologies evolve rapidly, bringing new features and best practices. Stay updated with the latest releases of Next.js and the React ecosystem to leverage any new enhancements that could benefit your form handling. Similarly, keeping an eye on evolving web standards and accessibility guidelines ensures your forms meet current expectations and requirements.
Conclusion: Beyond Form Handling
Mastering form handling in Next.js is about more than just collecting data efficiently; it's about creating engaging, accessible, and secure user experiences. By adopting best practices, leveraging modern tools and libraries, and staying attuned to user feedback and technological advancements, you can elevate your forms from simple data collection points to pivotal elements of your user's journey. Remember, the goal is to build forms that not only serve your needs but also delight and empower your users, fostering positive interactions with your application.
Conclusion
Handling forms in Next.js applications effectively requires understanding how to manage form state, validate inputs, handle submissions, and process data server-side. By following the practices outlined in this article, developers can create secure, efficient, and user-friendly forms in their Next.js applications. Whether you're building simple contact forms or complex data entry applications, these strategies will help ensure your forms are well-handled from front to back.